写一个可以winform保存窗体绘图并重新读取显示的程序,完成注释
时间: 2023-03-20 16:02:15 浏览: 107
好的,以下是一个简单的C# WinForm程序,它可以保存窗体上的绘图,并在需要时重新读取并显示出来。
```
using System;
using System.Drawing;
using System.Windows.Forms;
using System.IO;
namespace SaveAndLoadDrawings
{
public partial class Form1 : Form
{
private bool isDrawing = false;
private Point startPoint;
private Point endPoint;
private Bitmap bitmap;
public Form1()
{
InitializeComponent();
bitmap = new Bitmap(ClientSize.Width, ClientSize.Height);
pictureBox.Image = bitmap;
pictureBox.MouseMove += new MouseEventHandler(pictureBox_MouseMove);
pictureBox.MouseDown += new MouseEventHandler(pictureBox_MouseDown);
pictureBox.MouseUp += new MouseEventHandler(pictureBox_MouseUp);
}
private void pictureBox_MouseMove(object sender, MouseEventArgs e)
{
if (isDrawing)
{
endPoint = e.Location;
using (Graphics g = Graphics.FromImage(bitmap))
{
g.DrawLine(Pens.Black, startPoint, endPoint);
}
startPoint = endPoint;
pictureBox.Refresh();
}
}
private void pictureBox_MouseDown(object sender, MouseEventArgs e)
{
isDrawing = true;
startPoint = e.Location;
}
private void pictureBox_MouseUp(object sender, MouseEventArgs e)
{
isDrawing = false;
}
private void saveButton_Click(object sender, EventArgs e)
{
SaveFileDialog dialog = new SaveFileDialog();
dialog.Filter = "Bitmap files (*.bmp)|*.bmp";
if (dialog.ShowDialog() == DialogResult.OK)
{
using (FileStream fs = new FileStream(dialog.FileName, FileMode.Create))
{
bitmap.Save(fs, System.Drawing.Imaging.ImageFormat.Bmp);
}
}
}
private void loadButton_Click(object sender, EventArgs e)
{
OpenFileDialog dialog = new OpenFileDialog();
dialog.Filter = "Bitmap files (*.bmp)|*.bmp";
if (dialog.ShowDialog() == DialogResult.OK)
{
using (FileStream fs = new FileStream(dialog.FileName, FileMode.Open))
{
bitmap = new Bitmap(fs);
}
pictureBox.Image = bitmap;
pictureBox.Refresh();
}
}
}
}
```
这个程序使用一个pictureBox控件来显示绘图,当鼠标在pictureBox上移动时,会根据鼠标的位置绘制一条直线。当用户单击保存按钮时,程序会弹出一个SaveFileDialog来让用户选择保存的文件名和位置,并将当前窗体绘图保存为一个BMP文件。当用户单击加载按钮时,程序会弹出一个OpenFileDialog来让用户选择要加载的BMP文件,并将其显示在pictureBox上。
希望这个程序对您有帮助。
阅读全文
相关推荐
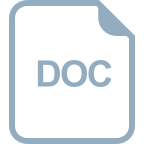
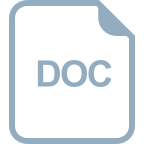
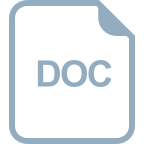
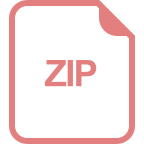
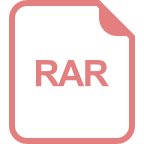
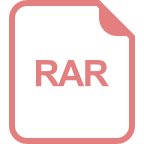
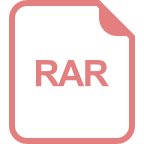
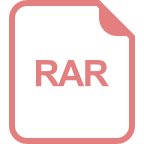
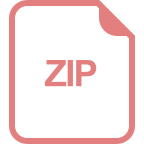
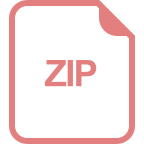
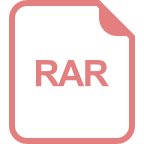
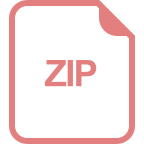
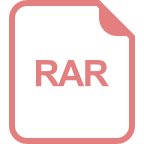
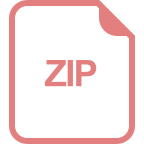
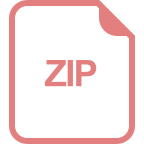
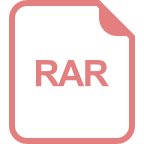
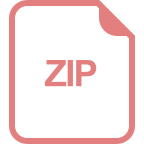
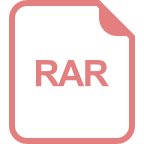