java上传照片到指定服务器
时间: 2023-08-09 16:07:06 浏览: 44
在Java中,你可以使用Apache HttpClient库来上传照片到指定的服务器。以下是一个简单的示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.entity.mime.content.FileBody;
import org.apache.http.impl.client.HttpClients;
import java.io.File;
public class PhotoUploader {
public static void main(String[] args) {
String serverUrl = "http://example.com/upload"; // 替换成实际的服务器URL
String filePath = "/path/to/photo.jpg"; // 替换成实际的照片文件路径
HttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(serverUrl);
File file = new File(filePath);
FileBody fileBody = new FileBody(file);
HttpEntity entity = MultipartEntityBuilder.create()
.addPart("file", fileBody)
.build();
httpPost.setEntity(entity);
try {
HttpResponse response = httpClient.execute(httpPost);
// 处理服务器响应
// ...
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上述示例中,你需要替换`serverUrl`为实际的服务器URL,`filePath`为照片的实际文件路径。然后使用`HttpClient`发送`HttpPost`请求,将照片文件作为`file`参数添加到`HttpEntity`中,并执行请求。你可以根据服务器的响应进行进一步的处理。
请注意,这只是一个简单的示例,实际应用中可能需要处理异常、添加其他参数等。此外,确保你已经包含了Apache HttpClient库的依赖。
相关推荐
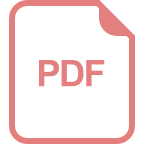
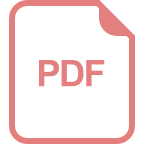
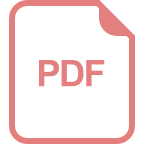














