Given 3 points on a 2D plane, design a function to do the following: determine whether 3 points lie on a straight line; if they do not lie on a straight line, draw a triangle with those points. (please import matplotlib) Note that the straight line or one of the triangle's edges may be perpendicular to the x-axis. Please take this possibility into account. The following shows the execution of your program.
时间: 2024-02-14 14:03:38 浏览: 25
Here is a Python function that takes in 3 points and determines whether they lie on a straight line or not. If they do not lie on a straight line, it uses Matplotlib to draw a triangle with those points:
```python
import matplotlib.pyplot as plt
def plot_triangle(p1, p2, p3):
# Check if the points lie on a straight line
if (p2[1]-p1[1])*(p3[0]-p2[0]) == (p3[1]-p2[1])*(p2[0]-p1[0]):
print('The points lie on a straight line.')
else:
# Create a figure and axis object
fig, ax = plt.subplots()
# Plot the points
ax.plot(p1[0], p1[1], 'bo')
ax.plot(p2[0], p2[1], 'bo')
ax.plot(p3[0], p3[1], 'bo')
# Plot the triangle
ax.plot([p1[0], p2[0]], [p1[1], p2[1]], 'r-')
ax.plot([p2[0], p3[0]], [p2[1], p3[1]], 'r-')
ax.plot([p3[0], p1[0]], [p3[1], p1[1]], 'r-')
# Set the x and y limits
ax.set_xlim(min(p1[0], p2[0], p3[0])-1, max(p1[0], p2[0], p3[0])+1)
ax.set_ylim(min(p1[1], p2[1], p3[1])-1, max(p1[1], p2[1], p3[1])+1)
# Show the plot
plt.show()
```
To use this function, simply call it with the 3 points as input:
```python
p1 = (1, 1)
p2 = (2, 3)
p3 = (4, 2)
plot_triangle(p1, p2, p3)
```
This will plot a triangle with the points `(1, 1)`, `(2, 3)`, and `(4, 2)`. If the points lie on a straight line, the function will print "The points lie on a straight line." instead of plotting the triangle.
相关推荐
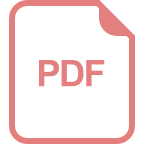
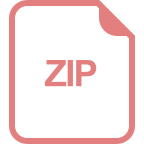













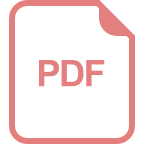
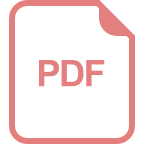