正则表达式匹配html标签
时间: 2023-08-31 09:10:48 浏览: 171
匹配HTML标签可以使用正则表达式。以下是一个简单示例:
```python
import re
html = '<p>This is a paragraph.</p><a href="https://www.google.com">Google</a>'
# 匹配HTML标签
pattern = re.compile(r'<.*?>')
matches = pattern.findall(html)
# 输出匹配结果
print(matches)
```
输出结果:
```
['<p>', '</p>', '<a href="https://www.google.com">', '</a>']
```
上述正则表达式`<.*?>`的含义是:
- `<`:匹配字符 "<"
- `.*?`:匹配任意字符,非贪婪模式
- `>`:匹配字符 ">"
因此,该正则表达式可以匹配所有的HTML标签。
相关问题
C#正则表达式匹配html标签
你可以使用以下的C#代码来匹配HTML标签:
```csharp
using System;
using System.Text.RegularExpressions;
class Program
{
static void Main()
{
string html = "<div><p>Hello, World!</p></div>";
// 正则表达式匹配HTML标签
string pattern = @"<[^>]+>";
MatchCollection matches = Regex.Matches(html, pattern);
foreach (Match match in matches)
{
Console.WriteLine(match.Value);
}
}
}
```
这段代码中,我们使用了 `<[^>]+>` 的正则表达式模式来匹配HTML标签。`<` 和 `>` 表示标签的开始和结束,`[^>]+` 表示匹配除了 `>` 之外的任意字符,`+` 表示匹配一个或多个前面的模式。
运行这段代码,它将输出匹配到的HTML标签:
```
<div>
<p>
</p>
</div>
```
希望能帮到你!如果还有其他问题,请随时提问。
正则判断文本为html标签,正则表达式匹配HTML标签和提取文本
判断文本是否为HTML标签的正则表达式:
```python
import re
def is_html_tag(text):
pattern = r'^<([a-z]+)([^<]+)*(?:>(.*)<\/\1>|\s+\/>)$'
return re.match(pattern, text) is not None
```
匹配HTML标签和提取文本的正则表达式:
```python
import re
def match_html_tag(text):
pattern = r'<([a-z]+)([^<]+)*(?:>(.*)<\/\1>|\s+\/>)'
return re.findall(pattern, text)
def extract_text_from_html(text):
pattern = r'<[^>]*>'
return re.sub(pattern, '', text)
```
其中,`match_html_tag`函数可以返回所有匹配到的HTML标签及其属性和内部文本,而`extract_text_from_html`函数可以提取出HTML文本中的纯文本内容。
阅读全文
相关推荐
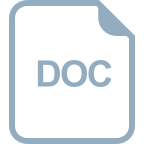
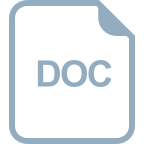
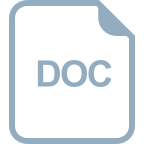
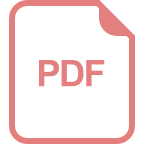
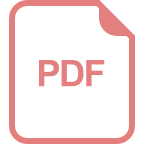







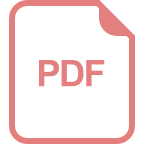
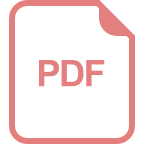
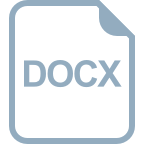

