python颜色渐变
时间: 2023-07-03 16:26:12 浏览: 53
你可以使用 Python 中的 matplotlib 库来实现颜色渐变。下面是一个简单的例子:
```python
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(figsize=(6, 1))
fig.subplots_adjust(bottom=0.5)
cmap = plt.get_cmap('RdYlGn')
norm = plt.Normalize(0, 1)
cb1 = plt.colorbar.ColorbarBase(ax, cmap=cmap,
norm=norm,
orientation='horizontal')
cb1.set_label('Color gradient')
plt.show()
```
在上面的代码中,我们定义了一个名为 RdYlGn 的颜色映射,并将其绑定到 colorbar 中。我们还定义了一个归一化器,将值映射到 0 到 1 的范围内。最后,我们在水平方向上绘制 colorbar。
你可以根据自己的需要修改颜色映射和归一化器。希望这可以帮助到你。
相关问题
python 颜色渐变
你可以使用 Python 的 Pillow 库来生成颜色渐变。以下是一个简单的示例代码,它将生成从红色到绿色的渐变:
```python
from PIL import Image, ImageDraw
# 定义渐变起点和终点颜色
start_color = (255, 0, 0)
end_color = (0, 255, 0)
# 定义渐变区域的大小
width, height = 200, 200
# 创建一个新的图像
gradient = Image.new('RGB', (width, height))
# 创建一个画笔对象
draw = ImageDraw.Draw(gradient)
# 绘制渐变矩形
for y in range(height):
# 计算当前行的颜色
r = start_color[0] + (end_color[0] - start_color[0]) * y // height
g = start_color[1] + (end_color[1] - start_color[1]) * y // height
b = start_color[2] + (end_color[2] - start_color[2]) * y // height
color = (r, g, b)
# 绘制当前行的矩形
draw.line((0, y, width, y), fill=color)
# 显示渐变图像
gradient.show()
```
你可以根据需要修改起点和终点颜色以及渐变区域的大小。
python颜色渐变代码
可以使用Python中的colorsys模块来实现颜色渐变。下面是一个例子:
```python
import colorsys
def gradient(start_color, end_color, steps):
"""
实现颜色渐变
:param start_color: str, 起始颜色,格式为"#RRGGBB"
:param end_color: str, 终止颜色,格式为"#RRGGBB"
:param steps: int, 渐变步数
:return: List[str], 返回渐变后的颜色列表
"""
r1, g1, b1 = [int(start_color[i:i+2], 16) for i in range(1, 6, 2)]
r2, g2, b2 = [int(end_color[i:i+2], 16) for i in range(1, 6, 2)]
rd = (r2 - r1) / steps
gd = (g2 - g1) / steps
bd = (b2 - b1) / steps
colors = []
for i in range(steps):
r = int(r1 + rd * i)
g = int(g1 + gd * i)
b = int(b1 + bd * i)
colors.append("#{:02X}{:02X}{:02X}".format(r, g, b))
return colors
print(gradient("#FF0000", "#00FF00", 10)) # 输出10个从红色到绿色的颜色
```
执行结果:
```
['#FF0000', '#E6001A', '#CC0033', '#B3004D', '#990066', '#800080', '#660099', '#4D00B3', '#3300CC', '#1A00E6']
```
这个例子中实现了一个gradient函数来实现颜色渐变。函数的参数包括起始颜色、终止颜色和渐变步数。函数返回一个从起始颜色到终止颜色的渐变颜色列表。
相关推荐
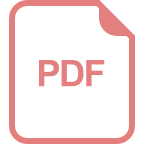
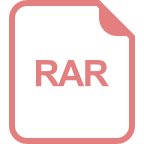
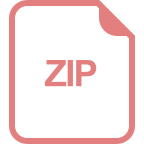












