用C语言编写STM32 通过SD更新程序
时间: 2023-05-30 08:04:59 浏览: 313
要实现通过SD卡更新STM32程序,需要以下步骤:
1. 初始化SD卡。使用HAL库提供的SD卡相关函数,初始化SD卡,检查SD卡是否插入,是否能够正常读取数据。
2. 读取程序文件。从SD卡中读取程序文件,将其存储到内存中。可以使用fread函数读取文件。
3. 擦除FLASH。使用HAL库提供的FLASH擦除函数,将STM32的FLASH全部擦除。
4. 将程序写入FLASH。将读取到的程序文件写入FLASH中。可以使用HAL库提供的FLASH编程函数。
5. 校验程序。通过校验程序的方式,确保程序写入FLASH中的正确性。可以使用CRC校验或者其他校验方式。
6. 跳转到新程序。使用函数指针,将程序的入口地址指向新程序的起始地址,然后跳转到新程序的入口。可以使用如下代码实现:
```
typedef void (*pFunction)(void);
pFunction Jump_To_Application;
// 跳转到新程序的入口
Jump_To_Application = (pFunction) (*(uint32_t*) (APPLICATION_ADDRESS+4));
// 跳转
Jump_To_Application();
```
其中,APPLICATION_ADDRESS为新程序在FLASH中的起始地址。
以上就是通过SD卡更新STM32程序的主要步骤。需要注意的是,为了避免程序在更新过程中出现问题,可以采用双备份机制,即将新程序写入两个不同的FLASH扇区,只有在新程序校验成功之后,才跳转到新程序的入口。
相关问题
编写stm32 SD卡 初始化 C语言
以下是一个简单的示例代码,演示如何在STM32上初始化SD卡:
```c
#include "stm32f4xx.h"
#include "stm32f4xx_sdio.h"
#include "stm32f4xx_rcc.h"
#include "stm32f4xx_gpio.h"
void SDIO_GPIO_Configuration(void);
void SDIO_Configuration(void);
void SDIO_Clock_Setup(uint8_t Div);
int main(void)
{
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOC | RCC_AHB1Periph_GPIOD, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SDIO, ENABLE);
SDIO_GPIO_Configuration();
SDIO_Configuration();
SDIO_Clock_Setup(0);
return 0;
}
void SDIO_GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* Configure the SDIO_D0-D3 Pins */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_8 | GPIO_Pin_9 | GPIO_Pin_10 | GPIO_Pin_11;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_OType = GPIO_OType_PP;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOC, &GPIO_InitStructure);
/* Configure the SDIO_CK Pin */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_12;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOC, &GPIO_InitStructure);
/* Configure the SDIO_CMD Pin */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_2;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOD, &GPIO_InitStructure);
/* Configure the SDIO Card Detect Pin */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOC, &GPIO_InitStructure);
/* Configure the SDIO Write Protect Pin */
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_1;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN;
GPIO_InitStructure.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOC, &GPIO_InitStructure);
/* Connect GPIO pins to SDIO peripheral */
GPIO_PinAFConfig(GPIOC, GPIO_PinSource8, GPIO_AF_SDIO);
GPIO_PinAFConfig(GPIOC, GPIO_PinSource9, GPIO_AF_SDIO);
GPIO_PinAFConfig(GPIOC, GPIO_PinSource10, GPIO_AF_SDIO);
GPIO_PinAFConfig(GPIOC, GPIO_PinSource11, GPIO_AF_SDIO);
GPIO_PinAFConfig(GPIOC, GPIO_PinSource12, GPIO_AF_SDIO);
GPIO_PinAFConfig(GPIOD, GPIO_PinSource2, GPIO_AF_SDIO);
}
void SDIO_Configuration(void)
{
SDIO_InitTypeDef SDIO_InitStructure;
SDIO_InitStructure.SDIO_ClockDiv = SDIO_ClockEdge_Rising;
SDIO_InitStructure.SDIO_ClockPowerSave = SDIO_ClockPowerSave_Disable;
SDIO_InitStructure.SDIO_BusWide = SDIO_BusWide_1b;
SDIO_InitStructure.SDIO_HardwareFlowControl = SDIO_HardwareFlowControl_Disable;
SDIO_InitStructure.SDIO_ClockDiv = 0x76;
SDIO_Init(&SDIO_InitStructure);
/* Enable the SDIO interrupts */
NVIC_InitTypeDef NVIC_InitStructure;
NVIC_InitStructure.NVIC_IRQChannel = SDIO_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
/* Enable the SDIO peripheral clock */
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_SDIO, ENABLE);
/* Enable the SDIO peripheral */
SDIO_Cmd(ENABLE);
}
void SDIO_Clock_Setup(uint8_t Div)
{
/* Set the SDIO clock frequency */
uint32_t SDIO_Clock = 48000000 / (2 * (Div + 2));
SDIO_SetPowerState(SDIO_PowerState_ON);
SDIO_ClockCmd(SDIO_Clock, ENABLE);
}
```
这里主要是初始化SDIO控制器和相关的GPIO引脚。需要注意的是,SD卡的初始化顺序是非常重要的,它需要按照特定的顺序来完成。因此,在实际应用中,需要根据具体的SD卡和控制器来进行设置。
阅读全文
相关推荐
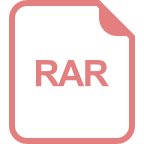
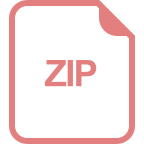
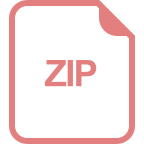
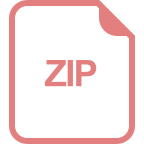
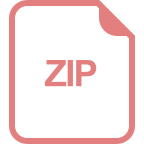
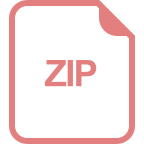
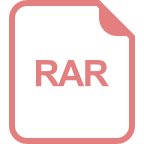
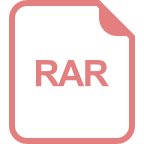
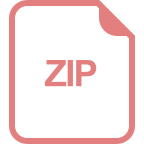
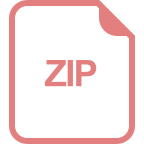
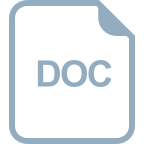
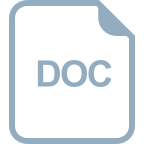
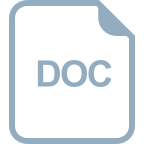
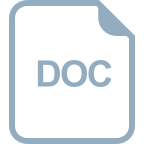
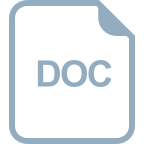