使用java代码检测指定springboot项目中使用的mysql地址,redis地址
时间: 2023-09-05 07:05:12 浏览: 139
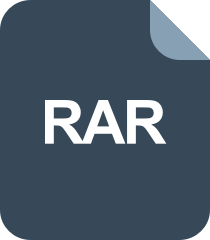
Java项目:OA自动化办公管理系统(java+SpringBoot+FreeMarker+Mysql+redis)
### 回答1:
要在Spring Boot应用程序中检测MySQL和Redis地址,可以使用以下代码:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.jdbc.DataSourceProperties;
import org.springframework.boot.autoconfigure.jdbc.DataSourceBuilder;
import org.springframework.boot.autoconfigure.data.redis.RedisProperties;
import org.springframework.boot.autoconfigure.data.redis.RedisConnectionFactoryBuilder;
import org.springframework.boot.autoconfigure.data.redis.RedisConnectionConfiguration;
import org.springframework.boot.autoconfigure.data.redis.RedisProperties.Pool;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.jedis.JedisConnectionFactory;
import javax.sql.DataSource;
public class ApplicationProperties {
@Autowired
private DataSourceProperties dataSourceProperties;
@Autowired
private RedisProperties redisProperties;
public String getMySqlUrl() {
return dataSourceProperties.getUrl();
}
public String getRedisUrl() {
Pool pool = redisProperties.getJedis().getPool();
RedisConnectionConfiguration redisConfig = new RedisConnectionConfiguration();
RedisConnectionFactory redisConnectionFactory = new JedisConnectionFactory(redisConfig);
redisConnectionFactory = new RedisConnectionFactoryBuilder(redisProperties).build();
return redisConnectionFactory.getHostName() + ":" + redisConnectionFactory.getPort();
}
}
```
在上面的代码中,我们使用`DataSourceProperties`和`RedisProperties`类获取数据源和Redis连接的配置。`DataSourceProperties`从Spring Boot的自动配置中获取数据源的配置,而`RedisProperties`获取Redis连接的配置。我们可以使用这些配置来获取MySQL和Redis的URL。
要使用上述代码,需要将其添加到Spring Boot应用程序的代码库中。可以将其作为单独的类添加到应用程序中,或将其添加到现有类中。要使用`ApplicationProperties`类,只需在其他类中将其注入为`@Autowired` bean,然后可以使用其方法来检索MySQL和Redis的URL。例如:
```java
@RestController
public class MyController {
@Autowired
private ApplicationProperties applicationProperties;
@GetMapping("/mysql-url")
public String getMySqlUrl() {
return applicationProperties.getMySqlUrl();
}
@GetMapping("/redis-url")
public String getRedisUrl() {
return applicationProperties.getRedisUrl();
}
}
```
在上面的代码中,我们创建了一个REST控制器,该控制器注入了`ApplicationProperties`类,并公开了两个端点:`/mysql-url`和`/redis-url`。当这些端点被调用时,它们将返回MySQL和Redis的URL,分别从`ApplicationProperties`类中检索。
### 回答2:
要使用Java代码检测指定Spring Boot项目中使用的MySQL地址和Redis地址,可以使用Java的反射机制来扫描项目的源代码并提取相关信息。
首先,通过反射获取指定项目的所有类文件。可以使用类似以下代码来实现:
```java
String projectPath = "指定项目的路径";
File file = new File(projectPath);
List<String> javaFilePaths = new ArrayList<>();
findJavaFiles(file, javaFilePaths);
List<Class<?>> classes = new ArrayList<>();
for (String javaFilePath : javaFilePaths) {
Class<?> clazz = Class.forName(javaFilePath.replace(projectPath + "/", "")
.replace("/", ".")
.replace(".java", ""));
classes.add(clazz);
}
// 扫描项目的源代码文件,将所有类的路径保存在javaFilePaths中
private static void findJavaFiles(File file, List<String> javaFilePaths) {
if (file.isDirectory()) {
File[] files = file.listFiles();
for (File subFile : files) {
findJavaFiles(subFile, javaFilePaths);
}
} else if (file.getName().endsWith(".java")) {
javaFilePaths.add(file.getPath());
}
}
```
接下来,遍历项目中的所有类,查找使用了MySQL和Redis的注解或配置。可以使用以下代码来检测MySQL和Redis地址:
```java
Set<String> mysqlAddresses = new HashSet<>();
Set<String> redisAddresses = new HashSet<>();
for (Class<?> clazz : classes) {
// 检查是否使用了MySQL的注解或配置
if (clazz.isAnnotationPresent(EnableJpaRepositories.class) || clazz.isAnnotationPresent(EntityScan.class) || clazz.isAnnotationPresent(EnableTransactionManagement.class)) {
ConfigurationProperties annotation = clazz.getAnnotation(ConfigurationProperties.class);
if (annotation != null && annotation.value().startsWith("spring.datasource")) {
String mysqlAddress = (String) AnnotationUtils.getValue(annotation);
mysqlAddresses.add(mysqlAddress);
}
}
// 检查是否使用了Redis的注解或配置
if (clazz.isAnnotationPresent(EnableRedisRepositories.class) || clazz.isAnnotationPresent(RedisHash.class)) {
ConfigurationProperties annotation = clazz.getAnnotation(ConfigurationProperties.class);
if (annotation != null && annotation.value().startsWith("spring.redis")) {
String redisAddress = (String) AnnotationUtils.getValue(annotation);
redisAddresses.add(redisAddress);
}
}
}
System.out.println("MySQL地址:");
for (String address : mysqlAddresses) {
System.out.println(address);
}
System.out.println("Redis地址:");
for (String address : redisAddresses) {
System.out.println(address);
}
```
通过运行以上代码,你可以获得指定Spring Boot项目中使用的MySQL地址和Redis地址。这里假设项目使用了Spring Data JPA和Spring Data Redis来与MySQL和Redis进行交互。如果项目使用其他方式进行数据库和缓存的操作,需要根据具体情况进行调整。
### 回答3:
可以通过使用Java代码来检测指定的Spring Boot项目中使用的MySQL地址和Redis地址。
首先,需要使用Java的反射机制来获取Spring Boot项目中配置文件中的数据库信息。可以通过加载项目中的配置文件,如application.properties或application.yml来获取MySQL地址和Redis地址的相关配置。
对于MySQL地址,可以使用如下代码示例来获取:
```java
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.Resource;
import org.springframework.util.StringUtils;
import java.io.IOException;
import java.util.Properties;
public class MySQLAddressDetector {
public static void main(String[] args) {
Properties properties = new Properties();
Resource resource = new ClassPathResource("application.properties");
try {
properties.load(resource.getInputStream());
String mysqlUrl = properties.getProperty("spring.datasource.url");
if (StringUtils.hasText(mysqlUrl)) {
System.out.println("MySQL地址:" + mysqlUrl);
} else {
System.out.println("未配置MySQL地址");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
对于Redis地址,可以使用如下代码示例来获取:
```java
import org.springframework.core.io.ClassPathResource;
import org.springframework.core.io.Resource;
import org.springframework.util.StringUtils;
import java.io.IOException;
import java.util.Properties;
public class RedisAddressDetector {
public static void main(String[] args) {
Properties properties = new Properties();
Resource resource = new ClassPathResource("application.properties");
try {
properties.load(resource.getInputStream());
String redisUrl = properties.getProperty("spring.redis.host");
if (StringUtils.hasText(redisUrl)) {
System.out.println("Redis地址:" + redisUrl);
} else {
System.out.println("未配置Redis地址");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这两个示例代码会加载项目中的配置文件,然后通过获取对应的属性值来获取MySQL地址和Redis地址。如果配置文件中没有对应的属性值,则输出相应的提示信息。
通过运行以上代码,即可检测到项目中使用的MySQL地址和Redis地址。
阅读全文
相关推荐
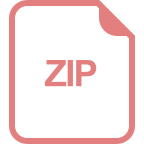
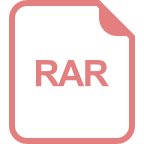















