C#用while循环,数组输出已知数的偶数
时间: 2023-03-13 13:00:49 浏览: 91
C# 中可以使用 while 循环来输出已知数字中的偶数,例如:int[] nums = {1,2,3,4,5}; int i = 0; while (i < nums.length) { if (nums[i] % 2 == 0) { System.out.println(nums[i]); } i++; } 这样可以输出 2 和 4。
相关问题
C#如何循环输出二维数组
要循环输出二维数组,你可以使用嵌套的 `for` 循环来遍历数组的每个元素并进行输出。以下是一个示例代码,演示了如何在 C# 中循环输出二维数组:
```csharp
int[,] array2D = {
{ 1, 2, 3 },
{ 4, 5, 6 },
{ 7, 8, 9 }
};
int numRows = array2D.GetLength(0);
int numCols = array2D.GetLength(1);
for (int i = 0; i < numRows; i++)
{
for (int j = 0; j < numCols; j++)
{
Console.Write(array2D[i, j] + " ");
}
Console.WriteLine();
}
```
上述代码创建了一个二维整数数组 `array2D`,然后使用 `GetLength` 方法获取数组的行数和列数。接下来,使用嵌套的 `for` 循环遍历每个元素,并使用 `Console.Write` 方法输出元素的值。在每行的末尾,使用 `Console.WriteLine` 方法打印换行符。这样,就可以按照行列的顺序输出二维数组中的所有元素。你可以根据需要修改数组内容和大小。
c#for循环生成数组
当然,C#中也可以使用循环来生成数组。可以使用如下代码来生成一个整型数组:
```
int[] arr = new int[10];
for (int i = 0; i < arr.Length; i++) {
arr[i] = i;
}
Console.WriteLine(string.Join(", ", arr));
```
这段代码使用 for 循环来遍历数组的每个索引,并将每个索引上的整数值设置为该索引的值。最后使用 `string.Join()` 方法将生成的数组转换为逗号分隔的字符串并输出。
相关推荐
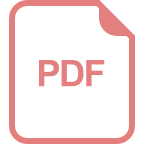












