python自定义函数
时间: 2023-07-07 20:12:33 浏览: 48
Python中可以使用def语句来定义自定义函数,语法如下:
```python
def function_name(parameters):
"""function_docstring"""
# function_body
return [expression]
```
其中,`function_name`为函数名,`parameters`为函数参数,可以是位置参数、默认参数、可变参数或关键字参数,多个参数之间用逗号隔开。`function_docstring`为函数文档字符串,用于描述函数的作用和参数说明。`function_body`为函数体,包含了函数的具体实现。`return`语句用于返回函数结果,可以省略,如果省略,则函数返回`None`。
例如,下面是一个简单的自定义函数,用于计算两个数的和:
```python
def add(x, y):
"""Return the sum of two numbers."""
result = x + y
return result
```
调用该函数可以使用如下语句:
```python
result = add(3, 5)
print(result) # 输出 8
```
在函数定义中,可以定义默认参数和可变参数,例如:
```python
def greet(name, greeting="Hello", *args):
"""Greet someone with a message."""
message = f"{greeting}, {name}!"
for arg in args:
message += f" {arg}"
return message
```
该函数包含了一个默认参数`greeting`和一个可变参数`args`,可以用如下方式进行调用:
```python
print(greet("Alice")) # 输出 "Hello, Alice!"
print(greet("Bob", "Hi")) # 输出 "Hi, Bob!"
print(greet("Charlie", "Hey", "how are you?")) # 输出 "Hey, Charlie! how are you?"
```
相关推荐
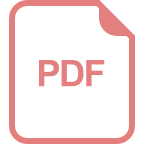
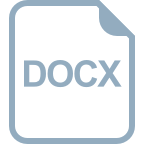
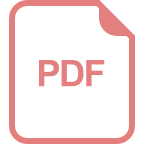












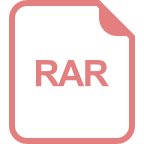