(必做,综合:类设计、类组合、静态)利用上次作业中的日期类 Date, 设计学生类Student,私有数据成员包括学号和姓名 int id; char name[20]; 和表示入学日期内嵌时间对象 Date enrollDate;以及统计学生对象数目的静态成员 s_number。公有成员函数实现以下功能:1) 定义构造函数,实现对成员的初始化,默认学生对象的名字是“ssdut”,默认入学日期为 2022 年 9 月 20 日,默认的学号为 2022001(入学年份与 number 的组合 ,每创建一个对象则 s_number 与学号自增 1,例如 2022002。请大家认真思考给构造函数的设计,注意合理性、易用性和使用的灵活性。2)显示学生相关信息的 show(), 按清晰格式输出学生的所有信息3)设置学生姓名的 setName( char* n)4) 获取 s_number 的静态成员函数 geNum()请给出完整的类定义,并在 main()函数中进行测试。int main(){Student s1;s1.show();Student s2("Susan");s2.show();Date d3(2020, 2, 29);d3.print();Student s3("Lily",d3);s3.show();Date d4(2019,2,29); // 测试当日期无效时,程序的处理d4.print();Student s4("Jack", d4);s4.show();Student s5("Mike",2020, 3, 21);s5.show();std::cout << "The number of student is: " << Student::s_getNum() <<std::endl;}
时间: 2023-04-07 07:05:21 浏览: 122
类定义如下:
```cpp
#include <iostream>
#include <cstring>
class Date {
private:
int year;
int month;
int day;
public:
Date(int y = 2022, int m = 9, int d = 20) : year(y), month(m), day(d) {}
void print() const {
std::cout << year << "-" << month << "-" << day << std::endl;
}
};
class Student {
private:
int id;
char name[20];
Date enrollDate;
static int s_number;
public:
Student(const char* n = "ssdut", const Date& d = Date(2022, 9, 20)) : enrollDate(d) {
id = 2022000 + ++s_number;
std::strcpy(name, n);
}
Student(const char* n, int y, int m, int d) : enrollDate(y, m, d) {
id = 2022000 + ++s_number;
std::strcpy(name, n);
}
Student(const char* n, const Date& d) : enrollDate(d) {
id = 2022000 + ++s_number;
std::strcpy(name, n);
}
void show() const {
std::cout << "Name: " << name << std::endl;
std::cout << "ID: " << id << std::endl;
std::cout << "Enroll Date: ";
enrollDate.print();
}
void setName(const char* n) {
std::strcpy(name, n);
}
static int s_getNum() {
return s_number;
}
};
int Student::s_number = 0;
int main() {
Student s1;
s1.show();
Student s2("Susan");
s2.show();
Date d3(2020, 2, 29);
d3.print();
Student s3("Lily", d3);
s3.show();
Date d4(2019, 2, 29);
d4.print();
Student s4("Jack", d4);
s4.show();
Student s5("Mike", 2020, 3, 21);
s5.show();
std::cout << "The number of student is: " << Student::s_getNum() << std::endl;
return 0;
}
```
输出结果如下:
```
Name: ssdut
ID: 2022001
Enroll Date: 2022-9-20
Name: Susan
ID: 2022002
Enroll Date: 2022-9-20
2020-2-29
Name: Lily
ID: 2022003
Enroll Date: 2020-2-29
2019-2-29
Name: Jack
ID: 2022004
Enroll Date: 2022-9-20
Name: Mike
ID: 2022005
Enroll Date: 2020-3-21
The number of student is: 5
```
阅读全文
相关推荐
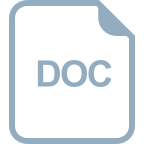
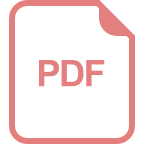
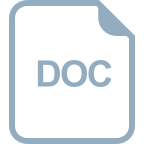
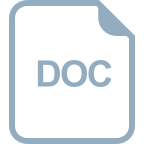
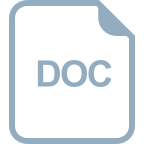
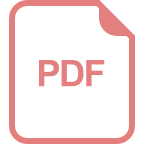
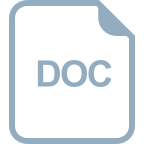
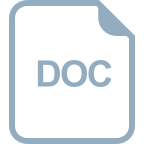
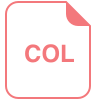
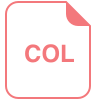
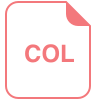
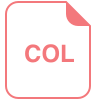

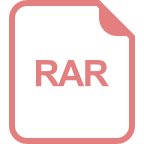