在js文件中获取vue3组件的实例
时间: 2023-09-27 18:06:16 浏览: 293
在 Vue 3 中,可以使用 `ref` 和 `setup` 函数来获取组件实例。
假设我们有以下组件:
```vue
<template>
<div>
<h1 ref="titleRef">{{ title }}</h1>
<button @click="increment">{{ count }}</button>
</div>
</template>
<script>
import { ref } from 'vue';
export default {
setup() {
const title = ref('Hello, World!');
const count = ref(0);
const increment = () => {
count.value++;
};
return {
title,
count,
increment,
};
},
};
</script>
```
要在 js 文件中获取组件实例,可以使用 `ref` 函数获取组件中的 DOM 元素,然后通过 `parent` 属性获取组件实例。例如:
```javascript
import { ref } from 'vue';
import MyComponent from './MyComponent.vue';
const myComponentRef = ref(null);
// 在组件渲染完成后,获取组件实例
setTimeout(() => {
const myComponentInstance = myComponentRef.value.$parent;
console.log(myComponentInstance.title.value); // 输出 "Hello, World!"
console.log(myComponentInstance.count.value); // 输出 0
myComponentInstance.increment(); // 调用组件中的方法
});
// 渲染组件
createApp({
components: {
MyComponent,
},
setup() {
return {
myComponentRef,
};
},
template: '<MyComponent ref="myComponentRef" />',
}).mount('#app');
```
在上面的例子中,我们首先使用 `ref` 函数创建了一个名为 `myComponentRef` 的引用变量。然后,在组件渲染完成后,我们通过 `myComponentRef.value.$parent` 获取了组件实例,并调用了组件中的方法。
阅读全文
相关推荐
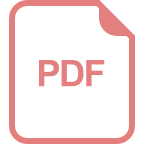
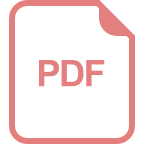
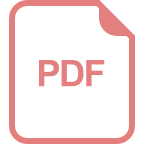















