写一段防止越权漏洞的java代码
时间: 2024-05-05 08:15:41 浏览: 18
为了防止越权漏洞,我们可以在Java代码中实现以下措施:
1. 权限验证:在代码中添加权限验证,只有具有特定权限的用户才能执行某些操作。例如,我们可以使用Spring Security框架来实现用户身份认证和授权。
2. 输入验证:在代码中添加输入验证,确保用户输入的数据符合预期的格式和类型。例如,我们可以使用正则表达式来验证用户输入的邮件地址或电话号码。
3. 数据过滤:在代码中添加数据过滤,确保只有特定的数据可以被访问和操作。例如,我们可以使用Hibernate框架来实现数据访问层,确保只有特定的实体可以被访问和操作。
4. 日志记录:在代码中添加日志记录,记录用户的访问和操作行为,以便后续审计和调查。例如,我们可以使用Log4j框架来实现日志记录。
下面是一个简单的Java代码示例,演示如何使用Spring Security框架来实现权限验证:
```
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Autowired
private CustomUserDetailsService userDetailsService;
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasAnyRole("USER", "ADMIN")
.antMatchers("/login").permitAll()
.and()
.formLogin()
.and()
.logout()
.logoutRequestMatcher(new AntPathRequestMatcher("/logout"))
.logoutSuccessUrl("/login");
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService).passwordEncoder(passwordEncoder());
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
```
在上面的代码中,我们定义了一个名为SecurityConfig的类,并使用@EnableWebSecurity注解启用Spring Security框架。然后,我们在configure方法中定义了访问规则和登录/登出配置。在configureGlobal方法中,我们将自定义的用户详细信息服务类和密码编码器添加到身份验证管理器中。最后,我们使用@Bean注解定义密码编码器的实例。
相关推荐
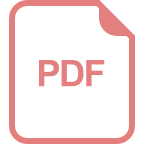














