非静态成员函数做thread
时间: 2023-04-08 11:03:54 浏览: 87
的入口函数时,需要注意哪些问题?
作为C知道,我可以回答这个问题。非静态成员函数做thread的入口函数时,需要注意以下几点:
1. 非静态成员函数需要通过对象来调用,因此需要先创建对象,然后将对象作为参数传递给thread的构造函数。
2. 非静态成员函数需要访问对象的成员变量和成员函数,因此需要保证对象的生命周期与线程的生命周期一致,否则可能会出现访问非法内存的问题。
3. 非静态成员函数不能直接作为线程的入口函数,需要通过一个静态的中间函数来调用非静态成员函数。
4. 非静态成员函数可能会访问共享资源,因此需要使用互斥量等同步机制来保证线程安全。
希望这些信息能对你有所帮助。
相关问题
pthread_create使用非静态成员函数
您好!对于使用pthread_create创建线程来说,它只能接受静态成员函数作为线程入口点。这是因为非静态成员函数需要一个隐含的this指针,而pthread_create只能接受一个普通函数作为线程入口点,没有办法传递额外的参数。
但是,您可以通过一些技巧来解决这个问题。一种方法是将非静态成员函数作为静态的全局函数的包装器,并在包装器中调用实际的非静态成员函数。
下面是一个示例代码:
```cpp
#include <pthread.h>
class MyClass {
public:
void myMemberFunction() {
// 非静态成员函数的实现
}
};
void* threadStart(void* arg) {
MyClass* obj = static_cast<MyClass*>(arg);
obj->myMemberFunction(); // 调用非静态成员函数
return nullptr;
}
int main() {
MyClass obj;
pthread_t thread;
pthread_create(&thread, NULL, &threadStart, &obj);
// ...
pthread_join(thread, NULL);
return 0;
}
```
在上面的示例中,我们定义了一个静态的全局函数threadStart作为线程的入口点,并通过参数arg传递了MyClass对象的地址。在threadStart函数中,我们将arg转换回MyClass指针,并调用实际的非静态成员函数myMemberFunction。
希望这个示例能对您有所帮助!如果您有任何其他问题,请随时提问。
C++类中的成员函数中如何使用pthread调用另一个带参数的非静态成员函数开启线程
在C++类中使用pthread调用另一个带参数的非静态成员函数开启线程,需要注意一些细节。以下是示例代码:
```c++
#include <pthread.h>
// 定义线程参数结构体
struct thread_data {
int arg1;
char* arg2;
MyClass* obj;
};
class MyClass {
public:
void myFunc() {
pthread_t thread;
struct thread_data data;
data.arg1 = 42;
data.arg2 = "hello";
data.obj = this;
// 创建线程
pthread_create(&thread, NULL, &MyClass::thread_func, (void*) &data);
}
void thread_func(int arg1, char* arg2) {
// do something with arg1 and arg2
}
};
```
在MyClass类的成员函数myFunc中,先定义了一个thread_data结构体,用于传递给线程函数的参数。其中,结构体中的obj成员变量保存了当前对象的指针,以便在线程函数中调用非静态成员函数。然后通过pthread_create函数创建了一个新的线程,并把thread_data结构体的地址传递给了静态成员函数thread_func。在thread_func中,通过结构体指针获取传递进来的参数,并调用非静态成员函数thread_func,并通过obj指针访问当前对象的成员变量等。注意,成员函数需要使用静态成员函数作为线程函数,并通过类名调用,而不是通过对象调用。
相关推荐
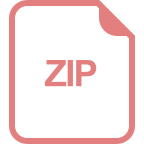
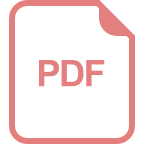
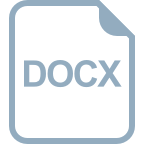








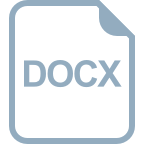
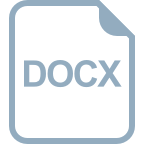
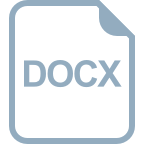
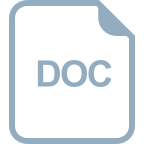
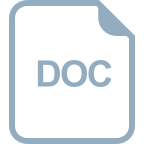