用c语言编写交换二叉树中所有节点的左右孩子的代码
时间: 2024-05-16 11:12:40 浏览: 83
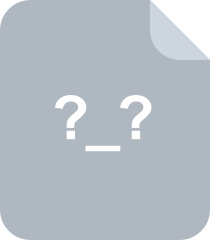
交换二叉树中所有结点的左右子树

以下是用 C 语言编写交换二叉树中所有节点的左右孩子的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树节点结构体
struct node {
int data;
struct node *left;
struct node *right;
};
// 交换二叉树中所有节点的左右孩子
void swapTree(struct node* root) {
// 判断节点是否为空
if (root == NULL) {
return;
}
// 递归交换左右孩子
struct node* temp = root->left;
root->left = root->right;
root->right = temp;
swapTree(root->left);
swapTree(root->right);
}
// 创建二叉树
struct node* createTree() {
int data;
printf("请输入节点数值(-1表示空节点):");
scanf("%d", &data);
if (data == -1) {
return NULL;
}
struct node* root = (struct node*) malloc(sizeof(struct node));
root->data = data;
root->left = createTree();
root->right = createTree();
return root;
}
// 前序遍历二叉树
void preOrder(struct node* root) {
if (root == NULL) {
return;
}
printf("%d ", root->data);
preOrder(root->left);
preOrder(root->right);
}
int main() {
struct node* root = createTree();
printf("交换前的二叉树(前序遍历):");
preOrder(root);
// 交换二叉树中所有节点的左右孩子
swapTree(root);
printf("\n交换后的二叉树(前序遍历):");
preOrder(root);
return 0;
}
```
代码解析:
1. 定义了一个二叉树节点的结构体,包含节点数值和左右孩子指针。
2. 定义了 `swapTree()` 函数,用于交换二叉树中所有节点的左右孩子。函数中先判断节点是否为空,如果不为空,就交换左右孩子,然后递归交换左右子树。
3. 定义了 `createTree()` 函数,用于创建二叉树。函数中先输入节点数值,如果节点数值为 `-1`,则表示为空节点,直接返回 `NULL`。否则,创建一个新节点,递归创建左右子树,并将左右孩子的指针赋给新节点。
4. 定义了 `preOrder()` 函数,用于前序遍历二叉树。函数中先判断节点是否为空,如果不为空,就先输出节点数值,然后递归遍历左右子树。
5. 在 `main()` 函数中,先创建二叉树,然后输出交换前的二叉树(前序遍历),调用 `swapTree()` 函数交换二叉树中所有节点的左右孩子,最后输出交换后的二叉树(前序遍历)。
阅读全文
相关推荐















