java实现从标准输入中输入两组整数(每行不超过20个整数,每组整数中元素不重复),求两组整数的异或集(合并两组整数,去掉在两组整数中都出现的整数后形成的集合)和交集(在两组整数中都出现的整数形成的集合),并按从大到小顺序排序输出。
时间: 2023-06-10 17:07:18 浏览: 252
可以使用Java中的Set集合来实现这个功能,具体步骤如下:
1. 从标准输入中读取两行整数,分别存储到两个Set集合中。
2. 创建一个新的Set集合,用于存储两组整数的异或集。遍历第一个Set集合,将其中的元素依次加入到新的Set集合中,然后遍历第二个Set集合,将其中不在新Set集合中的元素也加入到新Set集合中。
3. 创建一个新的Set集合,用于存储两组整数的交集。遍历第一个Set集合,将其中的元素依次判断是否在第二个Set集合中存在,如果存在,则加入到新的Set集合中。
4. 创建一个List集合,将新的Set集合中的元素依次加入到List集合中。
5. 使用Collections.sort()方法对List集合中的元素进行排序,按从大到小的顺序排序。
6. 遍历List集合,依次输出其中的元素。
下面是实现代码示例:
```java
import java.util.*;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 读取第一组整数
Set<Integer> set1 = new HashSet<>();
String[] nums1 = scanner.nextLine().split(" ");
for (String num : nums1) {
set1.add(Integer.parseInt(num));
}
// 读取第二组整数
Set<Integer> set2 = new HashSet<>();
String[] nums2 = scanner.nextLine().split(" ");
for (String num : nums2) {
set2.add(Integer.parseInt(num));
}
// 计算异或集
Set<Integer> xorSet = new HashSet<>();
xorSet.addAll(set1);
for (Integer num : set2) {
if (!xorSet.contains(num)) {
xorSet.add(num);
} else {
xorSet.remove(num);
}
}
// 计算交集
Set<Integer> intersectionSet = new HashSet<>();
for (Integer num : set1) {
if (set2.contains(num)) {
intersectionSet.add(num);
}
}
// 将异或集和交集合并到一个List集合中
List<Integer> result = new ArrayList<>();
result.addAll(xorSet);
result.addAll(intersectionSet);
// 对List集合中的元素按从大到小排序
Collections.sort(result, Collections.reverseOrder());
// 输出结果
for (Integer num : result) {
System.out.print(num + " ");
}
}
}
```
阅读全文
相关推荐









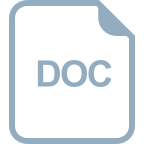

