编写一个C++程序:首先用头插法建立一个长度为10的带头结点的单链表,值任意;然后遍历此单链表,将10个元素的值在屏幕上输出。(简化起见,假设数据元素类型为int类型) 实现按位查找,并输出结果。
时间: 2024-09-19 19:04:03 浏览: 36
为了编写这个C++程序,你需要包含必要的头文件,定义节点结构,创建链表并填充元素,以及实现按位查找功能。下面是一个简单的示例:
```cpp
#include <iostream>
// 定义链表节点结构体
struct ListNode {
int data;
ListNode* next;
ListNode(int val) : data(val), next(nullptr) {}
};
// 使用头插法构建链表
ListNode* createLinkedList() {
ListNode* head = new ListNode(0); // 创建头节点
for (int i = 1; i <= 9; ++i) { // 遍历0到9
ListNode* newNode = new ListNode(i);
head->next = newNode;
head = newNode; // 更新头节点指向新节点
}
return head;
}
// 遍历链表并打印元素
void printList(ListNode* head) {
while (head != nullptr) {
std::cout << head->data << " ";
head = head->next;
}
std::cout << "\n";
}
// 按位查找函数(这里仅演示二进制表示)
bool bitSearch(ListNode* head, int target) {
int binaryTarget = 0; // 目标值的二进制表示
int index = 0;
// 将目标值转换为二进制
while (target > 0) {
binaryTarget |= (target % 2) << index++;
target >>= 1;
}
// 遍历链表并与二进制位进行比较
ListNode* current = head;
for (int i = 0; i < 10 && current != nullptr; ++i) {
if (binaryTarget & (1 << i)) {
std::cout << "Element at position " << i+1 << " is set.\n";
}
current = current->next;
}
return current == nullptr; // 如果未找到所有设定的位,则返回false
}
int main() {
ListNode* list = createLinkedList();
printList(list);
int targetValue = 5; // 示例目标值
if (bitSearch(list, targetValue)) {
std::cout << "All bits in the value " << targetValue << " are present in the list.\n";
} else {
std::cout << "Not all bits in the value " << targetValue << " are present in the list.\n";
}
delete list; // 清理内存
return 0;
}
```
在这个例子中,我们首先创建了一个长度为10的链表,然后遍历链表并打印每个节点的值。接着,我们实现了一个简单的按位查找算法,检查给定的目标值在链表中的哪些位置对应于1。如果所有目标位都存在于链表中,程序会输出相应的消息。
阅读全文
相关推荐
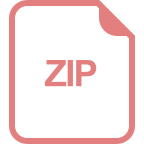
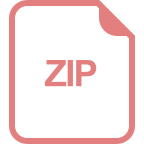
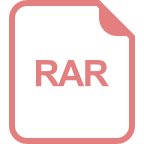





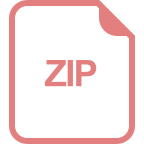
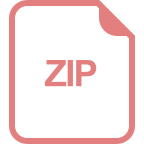
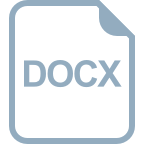
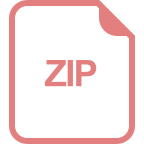