springboot连接elasticsearch
时间: 2023-08-16 07:09:22 浏览: 112
在Spring Boot中连接Elasticsearch,你需要完成以下几个步骤:
1. 添加Elasticsearch依赖:在你的项目的pom.xml文件中,添加以下依赖项:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
```
2. 配置Elasticsearch连接信息:在你的`application.properties`或者`application.yml`文件中,添加以下配置信息:
```properties
spring.data.elasticsearch.cluster-name=your-cluster-name
spring.data.elasticsearch.cluster-nodes=localhost:9300
```
3. 创建实体类:创建一个Java类来映射Elasticsearch中的文档。例如,如果你要存储一些产品信息,可以创建一个`Product`类,并使用注解来标记字段和文档的相关信息。
```java
@Document(indexName = "products", type = "_doc")
public class Product {
@Id
private String id;
private String name;
private String description;
// 省略构造函数、getter和setter方法
}
```
4. 创建Elasticsearch仓库接口:创建一个继承自`ElasticsearchRepository`的接口,用于对Elasticsearch进行CRUD操作。例如,对于`Product`实体类,可以创建一个`ProductRepository`接口。
```java
public interface ProductRepository extends ElasticsearchRepository<Product, String> {
// 可以自定义查询方法
}
```
5. 使用Elasticsearch操作数据:在你的服务类或控制器中,注入`ProductRepository`,并使用它来进行数据操作。例如:
```java
@Autowired
private ProductRepository productRepository;
// 保存一个产品
Product product = new Product("1", "手机", "这是一个手机");
productRepository.save(product);
// 根据ID查询一个产品
Optional<Product> optionalProduct = productRepository.findById("1");
```
以上是连接Elasticsearch的基本步骤,你可以根据自己的需求进一步扩展和定制。希望能对你有所帮助!如果有任何问题,请随时提问。
阅读全文
相关推荐
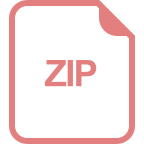
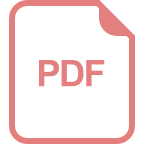
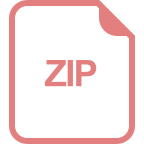
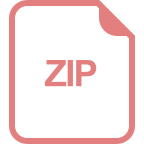











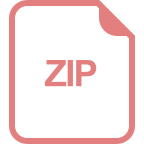
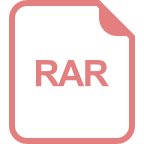