unity 等待下位机反馈再执行程序 ,多种写法
时间: 2024-12-12 13:30:08 浏览: 8
在Unity中,如果你需要等待某个“下位机”(通常是外部硬件设备、网络服务或者是另一个脚本发送的信号)的反馈后再继续执行程序,你可以使用几种不同的方法:
1. **Coroutine** (协程):Unity内置的Coroutine功能非常适合这种场景。你可以创建一个IEnumerator函数,里面包含`yield return new WaitForSeconds(realTimeSinceLastUpdate)` 或 `yield return new WaitForCompletion(waitForSignal)`这样的等待条件,直到收到预期的反馈信号才继续执行下一个yield。
```csharp
IEnumerator WaitForFeedback() {
while (!feedbackReceived) {
yield return new WaitForSeconds(0.1f); // 每隔0.1秒检查一次
}
// 当收到反馈,开始执行后续代码
}
```
2. **事件(Event)**:定义一个事件系统,当下位机准备好反馈时触发事件,然后在其他地方监听这个事件。
```csharp
public class DeviceManager : MonoBehaviour {
public event Action<DeviceStatus> FeedbackReceived;
void OnFeedbackReady(DeviceStatus status) {
FeedbackReceived?.Invoke(status);
}
}
// 使用处
public class YourScript : MonoBehaviour {
private DeviceManager deviceManager;
void Start() {
deviceManager = GetComponent<DeviceManager>();
deviceManager.FeedbackReceived += OnFeedbackReceivedHandler;
}
void OnFeedbackReceivedHandler(DeviceStatus status) {
// 执行后续操作
}
}
```
3. **异步任务(Async/Await)**:可以使用C#的async/await特性,配合Task.Run或Unity的`StartCoroutine`,编写非阻塞的等待代码。
```csharp
private async Task WaitForFeedbackAsync(Func<Task<bool>> isFeedbackReady) {
await isFeedbackReady();
// 反馈到达后执行
}
void Update() {
if (WaitForFeedback(isFeedbackReady)) {
// 开始执行后续操作
}
}
```
这里的`isFeedbackReady`是一个返回Task<bool>的方法,用于检查是否准备好反馈。
阅读全文
相关推荐
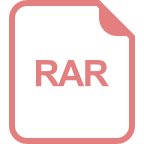
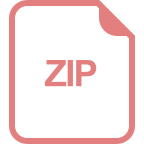

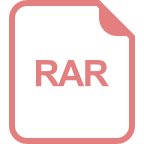
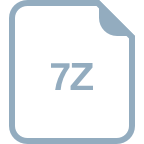
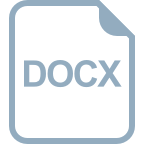
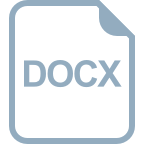
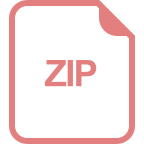
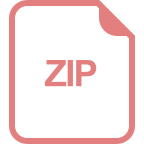
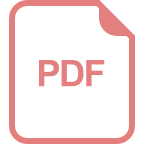
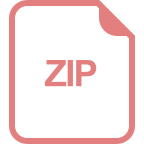
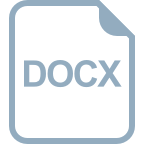
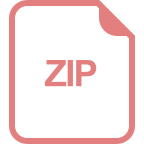