Scheduled怎么读
时间: 2023-11-17 22:03:52 浏览: 25
根据提供的引用内容,可以看出`@Scheduled`注解是用于定时任务的,可以通过读取配置文件中的cron表达式来设置定时任务的执行时间。下面是一个示例代码,演示如何使用`@Scheduled`注解和配置文件中的cron表达式来设置定时任务的执行时间:
```python
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class MyScheduledTask {
@Scheduled(cron = "${time.export.cron}")
public void performJob() throws Exception {
// do something
}
}
```
在上面的代码中,`@Scheduled`注解中的`cron`属性值`${time.export.cron}`表示从配置文件中读取名为`time.export.cron`的属性值作为cron表达式。这样就可以根据配置文件中的cron表达式来设置定时任务的执行时间。
相关问题
Scheduled用法
"Scheduled" 作为动词时,表示按计划安排或安排好某事物。它可以用来描述安排会议、活动、任务等。例如:
1. We have scheduled a meeting for tomorrow afternoon.
我们已经安排了明天下午的会议。)
2. The flight is scheduled to depart at 8:30 AM.
(航班计划在早上8:30起飞。)
3. The event is scheduled for next month.
(这个活动计划在下个月举行。)
"Scheduled" 作为形容词时,表示已经安排好的或按计划进行的。例如:
1. The train is running on its scheduled time.
(火车按预定时间运行。)
2. The maintenance work is scheduled for next week.
(维修工作计划在下周进行。)
3. The scheduled release date for the new product is in October.
(新产品的计划发布日期是在十月。)
希望这些例句能够帮助您理解 "Scheduled" 的用法。如果您还有其他问题,我很乐意为您解答!
Scheduled fixedRate
@Scheduled注解是Spring框架提供的一种定时任务调度的方式。其中fixedDelay和fixedRate都是用来设置任务执行的时间间隔的参数。
1. fixedDelay表示任务执行完成后,延时多长时间再次执行任务。例如,@Scheduled(fixedDelay=60*60*1000)表示每隔1小时执行一次任务。
2. fixedRate表示任务开始执行后,隔多长时间再次执行任务。例如,@Scheduled(fixedRate=60*60*1000)表示任务开始执行后,每隔1小时执行一次任务。
3. initialDelay表示任务首次执行的延时时间。例如,@Scheduled(initialDelay=5000, fixedRate=10000)表示任务首次延时5秒后执行,之后每隔10秒执行一次任务。
需要注意的是,@Scheduled注解需要与@EnableScheduling注解一起使用,才能使定时任务生效。
相关推荐
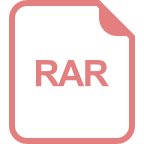
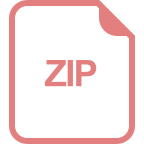












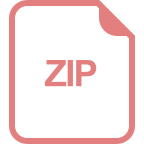