用java编写N个数据存放在数组A中,输入x,查找是否在A中存在,若存在 ,则输出第一次出现的位置号,否则输出-1
时间: 2024-09-21 20:12:35 浏览: 32
在Java中,你可以使用循环遍历数组的方式来实现这个功能。下面是一个简单的示例代码:
```java
public class ArraySearch {
public static int findPosition(int[] A, int x) {
// 检查数组是否为空
if (A == null || A.length == 0) {
return -1;
}
// 遍历数组
for (int i = 0; i < A.length; i++) {
// 如果找到目标值,返回当前位置
if (A[i] == x) {
return i;
}
}
// 如果整个数组都没有找到,返回-1
return -1;
}
public static void main(String[] args) {
int[] A = {1, 3, 5, 7, 9}; // 假设这是你要搜索的数组
int x = 5; // 输入的数值
int result = findPosition(A, x);
if (result != -1) {
System.out.println("元素 " + x + " 的位置是:" + result);
} else {
System.out.println("元素 " + x + " 不存在于数组中.");
}
}
}
```
在这个例子中,`findPosition` 方法接收一个整数数组 `A` 和一个目标值 `x`,然后通过遍历数组,寻找第一个等于 `x` 的元素。如果找到就返回它的索引,找不到就返回 `-1`。
阅读全文
相关推荐
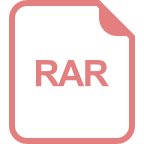
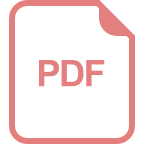
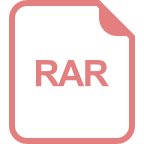















