vue3通过数组的长度 动态声明数组对应个数的ref
时间: 2024-11-17 11:30:45 浏览: 56
Vue3中,可以利用`ref`配合模板的动态特性来根据数组的长度动态声明对应个数的引用。`ref`是Vue官方提供的响应式数据包装器,它可以让你的数据在视图中自动更新。
假设我们有一个数组`items`,我们可以这样做:
```html
<template>
<div>
<div v-for="(item, index) in items" :key="index">
<input type="text" ref="itemRefs[index]" v-model="items[index]">
</div>
</div>
</template>
<script setup>
import { ref } from 'vue';
const items = ['A', 'B', 'C']; // 假设数组长度是3
// 使用计算属性动态创建ref
const itemRefs = computed(() => {
return items.map((value, index) => ref(value));
});
</script>
```
在这个例子中,`itemRefs`是一个计算属性,它会根据`items`数组的长度创建相应数量的`ref`实例。当你修改`items`数组时,对应的`ref`值也会随之改变,并在视图上实时更新。
相关问题
有一个数组里面有三个元素 分别对应三个列表 现在想实现一个删除功能 删除其中一个列表对应的数据数据也会发生变化 用Vue3怎么实现
在 Vue3 中,你可以通过响应式数据(ref 或者 computed)以及 watch 监听数组的变化来实现在一个数组中删除一个元素,同时影响其他关联的列表。这里是一个简单的例子:
首先,假设你有三个列表(list1, list2, list3),每个列表都是一个数组,存储着关联的数据,并且他们共享一个共同的索引:
```javascript
<template>
<div>
<button @click="deleteItem">删除</button>
<ul v-for="(item, index) in combinedLists" :key="index">
<li>{{ item }}</li>
</ul>
</div>
</template>
<script setup>
import { ref } from 'vue';
const list1 = ref([/*...*/]);
const list2 = ref([/*...*/]);
const list3 = ref([/*...*/]);
// 创建一个组合数组,例如:[list1.value, list2.value, list3.value]
let combinedLists = [list1, list2, list3];
function deleteItem(index) {
// 在这里删除对应的项,并更新所有相关的列表
combinedLists[index].splice(index, 1);
}
// 使用watch监听combinedLists的变化,当数组长度减小时触发回调
const lengthWatcher = watch(combinedLists.length, (newLength, oldLength) => {
if (newLength < oldLength) {
console.log('列表长度减少,发生了删除操作');
}
});
</script>
```
当你点击“删除”按钮时,会调用 `deleteItem` 函数,它会从 `combinedLists` 的相应位置删除一个元素,同时 `lengthWatcher` 将检测到数组长度变化,表明发生了删除操作。
注意,由于数组是响应式的,Vue会自动跟踪其变化并更新视图。
VUE v-for循环数组arr,div中显示对象中的某一值item.name,div横向排列,超出设定的长度时,div则自动换行,点击某一item.name时如何确定点击的元素是第几行的
要实现这个功能,你可以使用 Vue 的计算属性来计算每个 item 在数组中的索引以及所在的行数。具体操作步骤如下:
1. 在模板中使用 v-for 循环数组 arr,将每个 item 显示在一个 div 中,并绑定一个 click 事件。
```html
<template>
<div>
<div v-for="(item, index) in arr" :key="item.id" @click="handleClick(item)">
{{ item.name }}
</div>
</div>
</template>
```
2. 在计算属性中计算每个 item 在数组中的索引以及所在的行数。首先,需要知道每行能容纳的 item 的数量,可以根据容器的宽度和每个 item 的宽度来计算。然后,可以根据每个 item 在数组中的索引和每行能容纳的 item 的数量来计算该 item 所在的行数。
```javascript
computed: {
itemsPerRow() {
// 计算每行能容纳的 item 的数量
const containerWidth = this.$refs.container.clientWidth;
const itemWidth = this.$refs.item[0].clientWidth;
return Math.floor(containerWidth / itemWidth);
},
indexedArr() {
// 给每个 item 添加索引和行数属性
return this.arr.map((item, index) => {
const rowIndex = Math.floor(index / this.itemsPerRow);
return {
...item,
index,
rowIndex
};
});
}
}
```
3. 在 handleClick 方法中,可以通过事件对象的 target 属性获取点击的元素,然后遍历 indexedArr 数组,找到该元素对应的 item,并获取该 item 的 rowIndex 属性即可。
```javascript
methods: {
handleClick(item) {
const clickedElement = event.target;
const index = Array.from(this.$refs.item).indexOf(clickedElement);
const clickedItem = this.indexedArr[index];
console.log(clickedItem.rowIndex);
}
}
```
注意,这里需要使用 Array.from 将类数组对象转换为数组,以便使用 indexOf 方法来查找点击的元素在数组中的索引。同时,需要在每个 div 中添加一个 ref 属性,以便在计算属性中获取每个 item 的宽度。
阅读全文
相关推荐
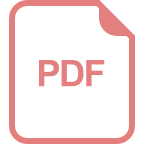
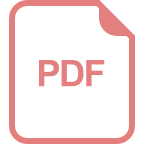
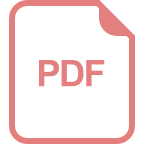
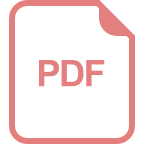
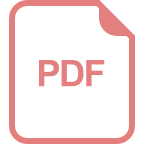
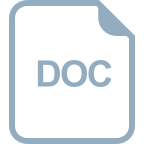
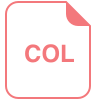
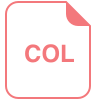
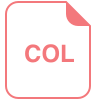







