Python分别编写凯撒密码的加密和解密程序
时间: 2024-11-27 10:18:36 浏览: 16
凯撒密码是一种简单的替换式密码,通过将明文中的每个字母按照字母表向前或向后移动固定的位置来进行加密。以下是Python编写简单凯撒密码加密和解密程序的例子:
**加密函数 (CaesarCipher_encrypt):**
```python
def caesar_cipher_encrypt(text, shift):
encrypted_text = ""
for char in text:
if char.isalpha():
shift_amount = shift % 26
if char.islower():
encrypted_text += chr((ord(char) - ord('a') + shift_amount) % 26 + ord('a'))
else:
encrypted_text += chr((ord(char) - ord('A') + shift_amount) % 26 + ord('A'))
else:
encrypted_text += char
return encrypted_text
```
**解密函数 (CaesarCipher_decrypt):**
```python
def caesar_cipher_decrypt(encrypted_text, shift):
return caesar_cipher_encrypt(encrypted_text, -shift)
```
**如何使用:**
```python
text_to_encrypt = "Hello, World!"
shift = 3
encrypted_text = caesar_cipher_encrypt(text_to_encrypt, shift)
decrypted_text = caesar_cipher_decrypt(encrypted_text, shift)
print(f"原始文本: {text_to_encrypt}")
print(f"加密后的文本: {encrypted_text}")
print(f"解密后的文本: {decrypted_text}")
```
阅读全文
相关推荐
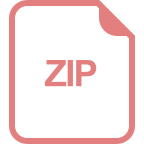
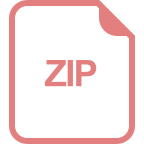
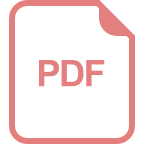












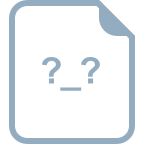
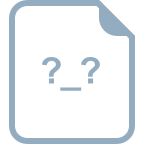
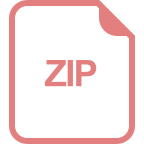