python生成3d图像
时间: 2023-07-12 20:13:00 浏览: 53
生成3D图像需要使用Python中的Matplotlib库中的mplot3d模块。以下是一个使用Matplotlib生成3D图像的简单示例:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 生成数据点
x = np.linspace(-5,5,50)
y = np.linspace(-5,5,50)
x, y = np.meshgrid(x, y)
z = np.sin(np.sqrt(x**2 + y**2))
# 创建3D图像对象
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# 绘制3D图像
ax.plot_surface(x, y, z)
# 设置坐标轴标签
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# 显示图像
plt.show()
```
在这个例子中,我们生成了一个由三个变量x、y和z组成的数据集。然后,我们使用Matplotlib的mplot3d模块来创建一个3D图像对象,并使用plot_surface函数绘制3D图像。最后,我们设置坐标轴标签并显示图像。
运行这段代码,你会看到一个基于sin函数的3D曲面图像。你可以通过更改数据集来创建不同的3D图像,以满足你的需求。
相关问题
blender python 生成3d
Blender Python是一种用于Blender 3D软件的编程语言,可以在Blender中自动化和扩展各种任务和功能。
要生成3D模型,您可以使用Blender Python API中的各种功能来创建和编辑对象,材质,纹理,光源等等。以下是一个简单的示例,演示如何使用Python脚本在Blender中创建一个立方体:
```python
import bpy
# 创建一个立方体对象
bpy.ops.mesh.primitive_cube_add(size=2)
# 将立方体移动到某个位置
bpy.context.active_object.location = (0, 0, 0)
# 创建一个新的材质
material = bpy.data.materials.new(name="CubeMaterial")
# 将材质分配给立方体
bpy.context.active_object.data.materials.append(material)
# 渲染图像
bpy.ops.render.render(write_still=True)
```
此代码将在Blender中创建一个2x2x2的立方体,并将其移动到原点。然后,它将创建一个新的材质并将其分配给立方体。最后,它会渲染图像并将其保存到磁盘。
这只是开始。您可以使用Blender Python API进行更复杂的任务,如动画,模拟,粒子系统等等。
python绘制3d函数图像
Python是一种功能强大的编程语言,可以用它来绘制3D函数图像。要绘制3D函数图像,我们可以使用Python中的matplotlib库和mplot3d工具包。
首先,我们需要安装matplotlib库。然后,我们可以使用以下代码来绘制一个简单的3D函数图像:
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 定义函数
def f(x, y):
return np.sin(np.sqrt(x**2 + y**2))
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
x, y = np.meshgrid(x, y)
z = f(x, y)
# 绘制图像
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot_surface(x, y, z, cmap='viridis')
# 设置图像标题和坐标轴标签
ax.set_title('3D Function Plot')
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# 显示图像
plt.show()
```
在这个例子中,我们定义了一个简单的函数f(x, y) = sin(√(x^2 + y^2)),然后使用numpy库生成了x和y的网格点,计算出z的值。接下来,我们用matplotlib的plot_surface方法绘制了3D函数图像,并设置了图像的标题和坐标轴标签。
当我们运行这段代码时,就会得到一个绘制了3D函数图像的窗口。
通过使用Python、matplotlib和mplot3d工具包,我们可以很容易地绘制出各种复杂的3D函数图像,帮助我们更好地理解和可视化数学函数。
相关推荐
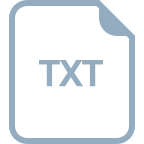
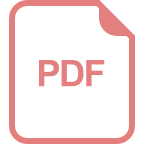












