生成一个pytorch可视化VGG特征图的代码
时间: 2023-08-31 18:39:00 浏览: 217
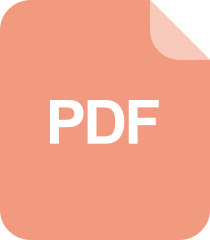
pytorch获取vgg16-feature层输出的例子
### 回答1:
可以使用以下代码来生成VGG特征图:import torch
import torchvision.models as models
import torchvision.transforms as transforms
from torch.autograd import Variable
import matplotlib.pyplot as plt# 载入VGG16模型
model = models.vgg16(pretrained=True)# 读入一张图片
img = Image.open('path_to_image')# 对图片做预处理
transform = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(
mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225]
)
])
img_t = transform(img)# 把图片做成一个batch
batch_t = torch.unsqueeze(img_t, 0)# 将VGG16模型设置为可训练
for parma in model.parameters():
parma.requires_grad = False # 为图片送入模型
out = model(Variable(batch_t))# 可视化VGG特征图
plt.figure(figsize=(14, 8))
for i in range(out.size()[1]):
ax = plt.subplot(8, 8, i + 1)
ax.imshow(out[0, i, :, :].data.numpy(), cmap='jet')
ax.axis('off')
plt.show()
### 回答2:
要生成一个pytorch可视化VGG特征图,需要进行以下步骤:
1. 导入必要的库和模块:
```python
import torch
import torch.nn as nn
import torchvision.models as models
import matplotlib.pyplot as plt
```
2. 定义VGG模型并加载预训练的权重:
```python
vgg = models.vgg16(pretrained=True)
```
3. 获取所需层的特征图:
```python
layer_name = 'features.29' # 这里选择VGG16的最后一个卷积层,可以根据需要调整layer_name
layer = vgg.features._modules[layer_name]
```
4. 创建一个向前传递的hook函数,用于获取特征图输出:
```python
target_output = None
def hook_fn(module, input, output):
global target_output
target_output = output
```
5. 注册hook函数到目标层:
```python
layer.register_forward_hook(hook_fn)
```
6. 前向传播输入并得到特征图:
```python
input_img = torch.randn(1, 3, 224, 224) # 这里随机生成一个输入图像,可以根据需要调整
_ = vgg(input_img) # 触发前向传播并生成特征图
```
7. 将特征图可视化:
```python
feature_map = target_output[0].detach().numpy() # 将特征图从Tensor转换为NumPy数组
plt.imshow(feature_map)
plt.show()
```
这样就可以生成并可视化VGG模型中指定层的特征图了。注意,代码中的图像尺寸、层的选择和其他参数可以根据需要进行调整。
### 回答3:
要生成PyTorch中VGG特征图的代码,需要首先导入必要的库和模块。代码如下所示:
```python
import torch
import torchvision.models as models
import cv2
import matplotlib.pyplot as plt
# 加载预训练的VGG模型
vgg = models.vgg16(pretrained=True)
vgg.eval()
# 选择指定层级的特征图
selected_layer = vgg.features[12]
# 加载并预处理图像
image = cv2.imread('image.jpg')
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
image = cv2.resize(image, (224, 224))
image = torch.from_numpy(image.transpose((2, 0, 1)))
image = image.float()
image /= 255.0
image = torch.unsqueeze(image, 0)
# 前向传播获取输出特征图
output = selected_layer(image)
# 转换输出特征图格式
output = output.data.squeeze()
output = output.numpy()
# 可视化特征图
plt.imshow(output, cmap='gray')
plt.axis('off')
plt.show()
```
以上代码首先加载了预训练的VGG16模型,并选择了第13层特征图(vgg.features[12])作为可视化对象。然后,加载和预处理了待处理的图像,将其转换为PyTorch张量。接下来,通过前向传播计算输出特征图。最后,使用matplotlib库将特征图可视化显示在屏幕上。请确保将图像文件路径替换为实际图像文件的路径。
阅读全文
相关推荐
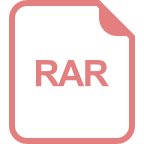
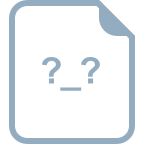










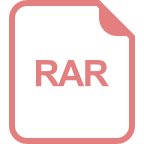
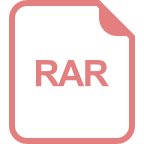
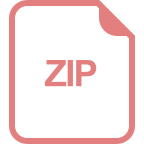
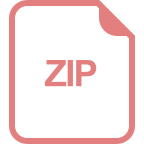
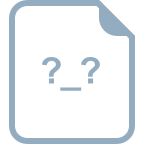
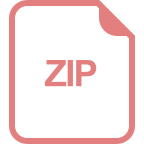