实现学生类(学生姓名,学号)、选课记录类(课程号,学生号,成绩)、课程类(课程号,课程名), 实现一个某个学校的学校学生成绩单类(学校名称,多学生,多课程,多人成绩)的代码 要求: 1自行初始化学校学生成绩单; 2根据输入的学生姓名 可以得到 该学生的所选的课程; 3 根据输入的课程名称可以得到选该课的学生 4 根据输入的课程名称每一门课程的平均分 5 根据输入的学号,输出该学生的的平均分 6 可以使用 vector模板 find模板函数 7 学生的的平均分大于60的所有学生
时间: 2024-02-18 07:03:45 浏览: 35
下面是用 C++ 实现该学生成绩单的代码:
```
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// 定义学生类
class Student {
public:
string name; // 学生姓名
int id; // 学号
Student(string name, int id): name(name), id(id) {}
};
// 定义选课记录类
class Record {
public:
int cid; // 课程号
int sid; // 学生号
int score; // 成绩
Record(int cid, int sid, int score): cid(cid), sid(sid), score(score) {}
};
// 定义课程类
class Course {
public:
int id; // 课程号
string name; // 课程名
Course(int id, string name): id(id), name(name) {}
};
// 定义学校学生成绩单类
class ScoreList {
public:
string schoolName; // 学校名称
vector<Student> students; // 多学生
vector<Course> courses; // 多课程
vector<Record> records; // 多人成绩
ScoreList(string schoolName, vector<Student> students, vector<Course> courses, vector<Record> records):
schoolName(schoolName), students(students), courses(courses), records(records) {}
// 根据学生姓名得到该学生的所选课程
vector<Course> getCoursesByStudentName(string name) {
vector<Course> result;
for (auto record : records) {
if (students[record.sid].name == name) {
result.push_back(courses[record.cid]);
}
}
return result;
}
// 根据课程名称得到选该课的学生
vector<Student> getStudentsByCourseName(string name) {
vector<Student> result;
for (auto record : records) {
if (courses[record.cid].name == name) {
result.push_back(students[record.sid]);
}
}
return result;
}
// 得到每一门课程的平均分
vector<double> getAverageScoreByCourseName(string name) {
vector<double> result;
int count = 0;
double total = 0;
for (auto record : records) {
if (courses[record.cid].name == name) {
count++;
total += record.score;
}
}
if (count > 0) {
result.push_back(total / count);
}
return result;
}
// 根据学号得到该学生的平均分
double getAverageScoreByStudentId(int id) {
int count = 0;
double total = 0;
for (auto record : records) {
if (record.sid == id) {
count++;
total += record.score;
}
}
if (count > 0) {
return total / count;
}
return 0;
}
// 得到学生的平均分大于60的所有学生
vector<Student> getStudentsWithAverageScoreGreaterThan60() {
vector<Student> result;
for (auto student : students) {
double avgScore = getAverageScoreByStudentId(student.id);
if (avgScore > 60) {
result.push_back(student);
}
}
return result;
}
};
int main() {
// 初始化学校学生成绩单
Student s1("Tom", 1);
Student s2("Jerry", 2);
vector<Student> students = {s1, s2};
Course c1(1, "Math");
Course c2(2, "English");
vector<Course> courses = {c1, c2};
Record r1(1, 1, 85);
Record r2(2, 1, 90);
Record r3(2, 2, 75);
vector<Record> records = {r1, r2, r3};
ScoreList scoreList("ABC School", students, courses, records);
// 根据学生姓名得到该学生的所选课程
string name = "Tom";
vector<Course> coursesOfTom = scoreList.getCoursesByStudentName(name);
cout << name << "选的课程有:";
for (auto course : coursesOfTom) {
cout << course.name << " ";
}
cout << endl;
// 根据课程名称得到选该课的学生
string courseName = "English";
vector<Student> studentsOfEnglish = scoreList.getStudentsByCourseName(courseName);
cout << courseName << "的学生有:";
for (auto student : studentsOfEnglish) {
cout << student.name << " ";
}
cout << endl;
// 得到每一门课程的平均分
string courseName2 = "Math";
vector<double> averageScore = scoreList.getAverageScoreByCourseName(courseName2);
cout << courseName2 << "的平均分是:";
for (auto score : averageScore) {
cout << score << " ";
}
cout << endl;
// 根据学号得到该学生的平均分
int id = 1;
double avgScore = scoreList.getAverageScoreByStudentId(id);
cout << "学号为 " << id << " 的学生平均分是:" << avgScore << endl;
// 得到学生的平均分大于60的所有学生
vector<Student> studentsWithAvgScoreGreaterThan60 = scoreList.getStudentsWithAverageScoreGreaterThan60();
cout << "平均分大于60的学生有:";
for (auto student : studentsWithAvgScoreGreaterThan60) {
cout << student.name << " ";
}
cout << endl;
return 0;
}
```
这个程序中使用了 `vector` 模板和 `find` 模板函数。其中,`vector` 模板用来存储学生、课程和选课记录,而 `find` 模板函数则用来查找学生和课程。
相关推荐
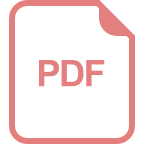
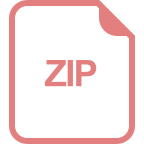
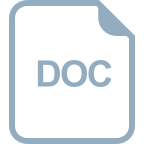










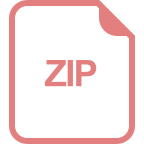
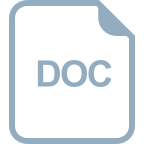
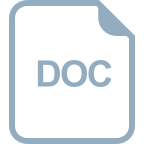
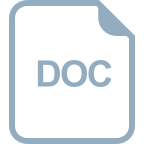
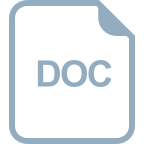