A star算法在有向图中代码matlab
时间: 2024-02-09 20:07:50 浏览: 120
这里是A*算法在有向图中的Matlab代码,它实现了从起点到终点的最短路径搜索:
```
function [path, cost] = A_star(start, goal, edges, weights, h)
% start: 起点的编号
% goal: 终点的编号
% edges: 边的列表,每一行是一个有向边的起点和终点编号
% weights: 边的权重,每一个元素是一个非负实数,表示该边的代价
% h: 启发式函数,输入一个节点编号,输出该节点到终点的估计距离
% 初始化数据结构
n = length(h); % 图中节点的数量
f = Inf(n, 1); % 从起点到该节点的路径代价估计
g = Inf(n, 1); % 从起点到该节点的实际路径代价
parent = zeros(n, 1); % 该节点在路径上的前一个节点
open_list = [start]; % 待扩展的节点列表
% 设置起点的代价
f(start) = h(start);
g(start) = 0;
% 开始搜索
while ~isempty(open_list)
% 选择代价最小的节点进行扩展
[min_f, current] = min(f(open_list));
if current == goal
% 找到了终点,构造路径并返回
path = [goal];
while parent(path(1)) ~= 0
path = [parent(path(1))), path];
end
path = [start, path];
cost = g(goal);
return;
end
% 从待扩展列表中移除当前节点
open_list(current) = [];
% 扩展当前节点
neighbors = find(edges(:, 1) == current);
for i = 1:length(neighbors)
neighbor = edges(neighbors(i), 2);
tentative_g = g(current) + weights(neighbors(i));
if tentative_g < g(neighbor)
% 更新节点代价
parent(neighbor) = current;
g(neighbor) = tentative_g;
f(neighbor) = g(neighbor) + h(neighbor);
% 将新扩展的节点加入待扩展列表中
if ~ismember(neighbor, open_list)
open_list = [open_list, neighbor];
end
end
end
end
% 如果搜索失败,则返回空路径和代价
path = [];
cost = Inf;
end
```
需要注意的是,该代码中的`h`函数是一个启发式函数,它需要根据具体情况进行定义。在实际应用中,通常可以使用曼哈顿距离等简单的函数作为启发式函数。
阅读全文
相关推荐
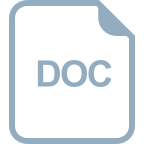
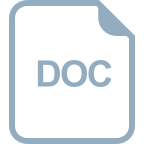
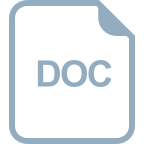
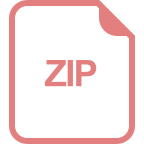
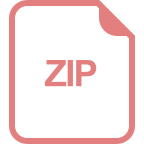
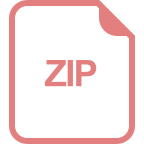
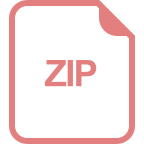
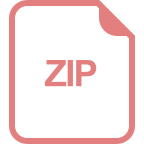
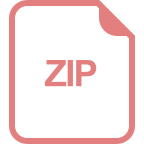
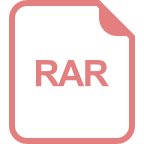
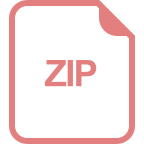
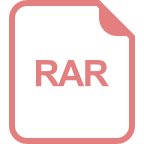
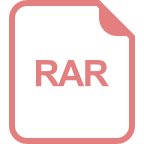
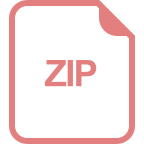
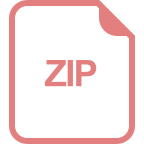
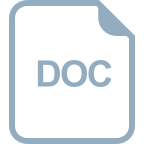
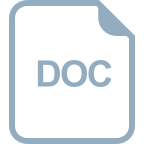
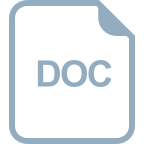
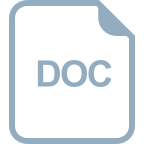