写一份倒排序索引的实现的报告及其完整代码
时间: 2024-01-24 07:16:46 浏览: 75
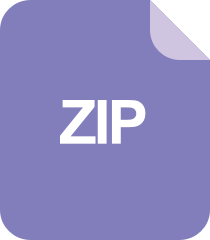
hadoop倒排索引实现 完整代码+报告

倒排序索引(Inverted Index)是一种用于文本检索的数据结构。它将每个单词与出现该单词的文档ID列表相关联。倒排序索引通常用于搜索引擎中,用于快速检索文档。
倒排序索引的实现需要两个步骤:首先,需要将文档分解为单词;其次,需要将每个单词与出现该单词的文档ID相关联。
以下是倒排序索引的实现代码:
```python
from collections import defaultdict
class InvertedIndex:
def __init__(self):
self.index = defaultdict(list)
def add_document(self, doc_id, text):
words = text.split()
for word in words:
self.index[word].append(doc_id)
def search(self, query):
words = query.split()
result = set(self.index[words[0]])
for word in words[1:]:
result &= set(self.index[word])
return list(result)
```
在这个实现中,我们使用了Python标准库中的defaultdict来创建倒排序索引。我们还定义了两个方法:add_document和search。
add_document方法接受一个文档ID和文本,将文本分解为单词,并将每个单词与出现该单词的文档ID相关联。
search方法接受一个查询,将查询分解为单词,并查找包含所有这些单词的文档ID。我们使用set和&运算符来实现这个操作。
以下是使用这个实现来构建倒排序索引的示例:
```python
index = InvertedIndex()
index.add_document(1, "The quick brown fox jumps over the lazy dog")
index.add_document(2, "The brown fox is quick and the blue dog is lazy")
index.add_document(3, "The quick blue fox jumps over the lazy brown dog")
result = index.search("quick brown")
print(result) # [1, 3]
```
在这个示例中,我们首先创建了一个InvertedIndex对象。然后,我们使用add_document方法添加了三个文档。最后,我们使用search方法搜索包含单词“quick”和“brown”的文档,并得到了包含文档ID 1和3的结果。
这个实现可以用于小型文本集合的快速搜索,但在处理大型文本集合时可能会遇到性能问题。为了优化性能,我们可以使用更高级的数据结构,例如倒排序文件(Inverted File)或倒排序表(Inverted Table)。
阅读全文
相关推荐
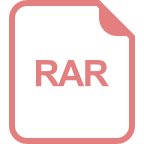

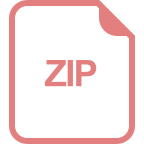
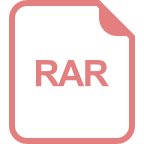
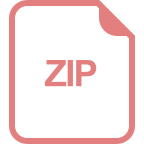
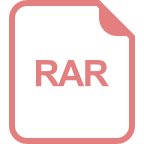
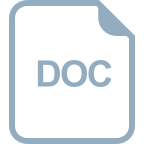
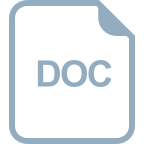
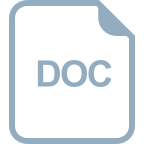
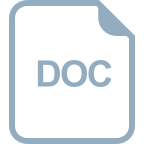
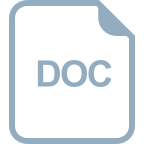
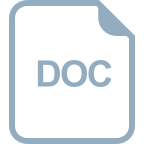
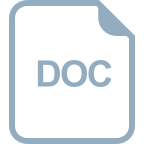
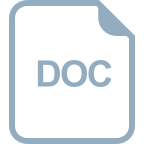
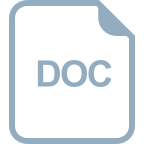
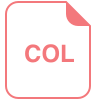
