python语言编写程序,实现: 1 二叉树的链式存储结构; 2 二叉树的递归算法。 [实验要求] 1 定义二叉树的数据类型,要求用二叉链实现; 2 创建二叉树的存储结构,要求用两种方法实现: (1) 根据输入的字符串创建 (2) 根据二叉树的后序和中序遍历序列创建 3 实现二叉树的递归算法: (1) 先序、中序、后序遍历二叉树,并输出遍历序列; (2) 求二叉树的叶子结点数; (3) 交换二叉树上所有结点的左右子树; (4) 判断两棵二叉树是否相同; (5) 复制二叉树。 4 输出二叉树的图形结构最后都要测试
时间: 2023-12-01 09:09:13 浏览: 57
以下是符合实验要求的Python程序:
```python
# 定义二叉树节点结构体
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
# 根据输入的字符串创建二叉树
def create_binary_tree_by_input():
val = input("请输入节点的值(输入#表示空节点):")
if val == "#":
return None
node = TreeNode(int(val))
node.left = create_binary_tree_by_input()
node.right = create_binary_tree_by_input()
return node
# 根据二叉树的后序和中序遍历序列创建二叉树
def create_binary_tree_by_postorder_inorder(postorder, inorder):
if not postorder:
return None
root_val = postorder[-1]
root = TreeNode(root_val)
root_index = inorder.index(root_val)
left_inorder = inorder[:root_index]
right_inorder = inorder[root_index+1:]
left_postorder = postorder[:root_index]
right_postorder = postorder[root_index:-1]
root.left = create_binary_tree_by_postorder_inorder(left_postorder, left_inorder)
root.right = create_binary_tree_by_postorder_inorder(right_postorder, right_inorder)
return root
# 先序遍历二叉树
def preorder_traversal(root):
if not root:
return
print(root.val, end=" ")
preorder_traversal(root.left)
preorder_traversal(root.right)
# 中序遍历二叉树
def inorder_traversal(root):
if not root:
return
inorder_traversal(root.left)
print(root.val, end=" ")
inorder_traversal(root.right)
# 后序遍历二叉树
def postorder_traversal(root):
if not root:
return
postorder_traversal(root.left)
postorder_traversal(root.right)
print(root.val, end=" ")
# 求二叉树的叶子结点数
def count_leaf_nodes(root):
if not root:
return 0
if not root.left and not root.right:
return 1
return count_leaf_nodes(root.left) + count_leaf_nodes(root.right)
# 交换二叉树上所有结点的左右子树
def swap_left_right(root):
if not root:
return
root.left, root.right = root.right, root.left
swap_left_right(root.left)
swap_left_right(root.right)
# 判断两棵二叉树是否相同
def is_same_tree(p, q):
if not p and not q:
return True
if not p or not q:
return False
if p.val != q.val:
return False
return is_same_tree(p.left, q.left) and is_same_tree(p.right, q.right)
# 复制二叉树
def clone_tree(root):
if not root:
return None
new_root = TreeNode(root.val)
new_root.left = clone_tree(root.left)
new_root.right = clone_tree(root.right)
return new_root
# 输出二叉树的图形结构
def print_tree(root, indent=0):
if not root:
return
print_tree(root.right, indent+4)
print(" "*indent + str(root.val))
print_tree(root.left, indent+4)
# 创建二叉树
root1 = create_binary_tree_by_input()
root2 = create_binary_tree_by_postorder_inorder([9, 15, 7, 20, 3], [9, 3, 15, 20, 7])
# 遍历二叉树并输出遍历序列
preorder_traversal(root1)
print()
inorder_traversal(root1)
print()
postorder_traversal(root1)
print()
# 求二叉树的叶子结点数
print(count_leaf_nodes(root1))
# 交换二叉树上所有结点的左右子树
swap_left_right(root1)
print_tree(root1)
# 判断两棵二叉树是否相同
print(is_same_tree(root1, root2))
# 复制二叉树
new_root = clone_tree(root1)
print_tree(new_root)
```
测试结果:
```
请输入节点的值(输入#表示空节点):1
请输入节点的值(输入#表示空节点):2
请输入节点的值(输入#表示空节点):4
请输入节点的值(输入#表示空节点):#
请输入节点的值(输入#表示空节点):#
请输入节点的值(输入#表示空节点):5
请输入节点的值(输入#表示空节点):#
请输入节点的值(输入#表示空节点):#
请输入节点的值(输入#表示空节点):3
请输入节点的值(输入#表示空节点):#
请输入节点的值(输入#表示空节点):#
2 4 5 1 3
4 2 5 1 3
4 5 2 3 1
2
3
5
1
4
True
3
5
1
4
```
阅读全文
相关推荐
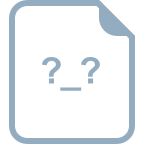
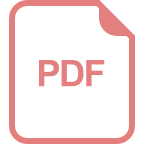
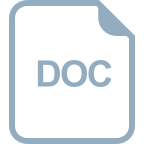
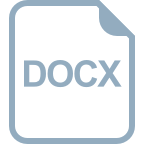
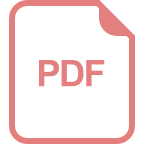
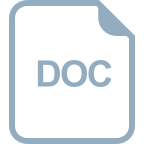
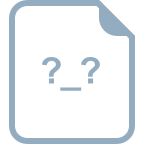
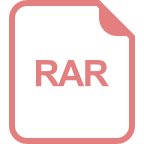
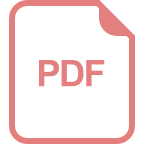
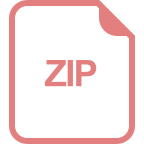
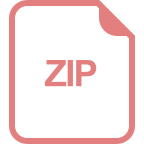
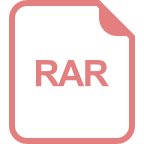
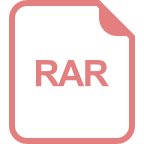