用python数据结构顺序表编辑通讯录
时间: 2023-07-12 15:45:45 浏览: 71
好的,下面是一个简单的用Python实现的通讯录管理程序,使用顺序表作为数据结构:
```python
class Contact:
def __init__(self, name, phone, email):
self.name = name
self.phone = phone
self.email = email
class AddressBook:
def __init__(self):
self.contacts = []
def add_contact(self, contact):
self.contacts.append(contact)
def remove_contact(self, name):
for contact in self.contacts:
if contact.name == name:
self.contacts.remove(contact)
break
def find_contact(self, name):
for contact in self.contacts:
if contact.name == name:
return contact
return None
def edit_contact(self, name, phone, email):
for contact in self.contacts:
if contact.name == name:
contact.phone = phone
contact.email = email
break
def display_contacts(self):
for contact in self.contacts:
print("Name:", contact.name)
print("Phone:", contact.phone)
print("Email:", contact.email)
print()
def main():
address_book = AddressBook()
while True:
print("1. Add contact")
print("2. Remove contact")
print("3. Find contact")
print("4. Edit contact")
print("5. Display contacts")
print("6. Quit")
choice = int(input("Enter your choice: "))
if choice == 1:
name = input("Enter name: ")
phone = input("Enter phone: ")
email = input("Enter email: ")
contact = Contact(name, phone, email)
address_book.add_contact(contact)
print("Contact added.")
elif choice == 2:
name = input("Enter name: ")
address_book.remove_contact(name)
print("Contact removed.")
elif choice == 3:
name = input("Enter name: ")
contact = address_book.find_contact(name)
if contact:
print("Name:", contact.name)
print("Phone:", contact.phone)
print("Email:", contact.email)
else:
print("Contact not found.")
elif choice == 4:
name = input("Enter name: ")
phone = input("Enter phone: ")
email = input("Enter email: ")
address_book.edit_contact(name, phone, email)
print("Contact updated.")
elif choice == 5:
address_book.display_contacts()
elif choice == 6:
break
else:
print("Invalid choice. Try again.")
if __name__ == '__main__':
main()
```
这个程序中,`Contact` 类表示一个通讯录条目,包含姓名、电话和电子邮件等信息。`AddressBook` 类表示整个通讯录,包含了添加、删除、查找、编辑和显示通讯录条目的方法。在程序运行时,会循环显示一个菜单,用户可以选择需要执行的操作。每个操作都会调用 `AddressBook` 类中对应的方法来完成相应的操作。
相关推荐
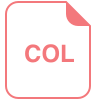
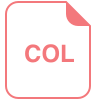
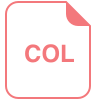
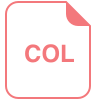
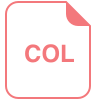









