sqlite3 C++ 查询数据
时间: 2023-08-28 11:07:50 浏览: 150
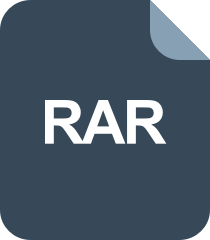
C++项目中使用到sqlite处理数据

要查询SQLite3数据库中的数据,可以使用以下步骤:
1. 打开数据库连接:
```c
sqlite3 *db;
int rc = sqlite3_open("mydatabase.db", &db);
if (rc != SQLITE_OK) {
fprintf(stderr, "Cannot open database: %s\n", sqlite3_errmsg(db));
sqlite3_close(db);
return 1;
}
```
2. 构造查询语句:
```c
const char *sql = "SELECT * FROM mytable";
```
这里查询了名为"mytable"的表中的所有数据。
3. 准备查询语句:
```c
sqlite3_stmt *stmt;
rc = sqlite3_prepare_v2(db, sql, -1, &stmt, 0);
if (rc != SQLITE_OK) {
fprintf(stderr, "Failed to prepare statement: %s\n", sqlite3_errmsg(db));
sqlite3_close(db);
return 1;
}
```
4. 执行查询语句:
```c
rc = sqlite3_step(stmt);
while (rc == SQLITE_ROW) {
// 处理查询结果
int id = sqlite3_column_int(stmt, 0);
const unsigned char *name = sqlite3_column_text(stmt, 1);
double price = sqlite3_column_double(stmt, 2);
// 输出结果
printf("id = %d, name = %s, price = %.2f\n", id, name, price);
// 继续查询下一行
rc = sqlite3_step(stmt);
}
```
这里使用了sqlite3_step()函数来执行查询语句并返回查询结果,如果返回值是SQLITE_ROW,则表示还有一行数据需要处理。在处理数据时,可以使用sqlite3_column_*()函数来获取每一列的值。
5. 关闭查询语句和数据库连接:
```c
sqlite3_finalize(stmt);
sqlite3_close(db);
```
完整的查询数据示例代码如下:
```c
#include <stdio.h>
#include <sqlite3.h>
int main(void) {
sqlite3 *db;
int rc = sqlite3_open("mydatabase.db", &db);
if (rc != SQLITE_OK) {
fprintf(stderr, "Cannot open database: %s\n", sqlite3_errmsg(db));
sqlite3_close(db);
return 1;
}
const char *sql = "SELECT * FROM mytable";
sqlite3_stmt *stmt;
rc = sqlite3_prepare_v2(db, sql, -1, &stmt, 0);
if (rc != SQLITE_OK) {
fprintf(stderr, "Failed to prepare statement: %s\n", sqlite3_errmsg(db));
sqlite3_close(db);
return 1;
}
rc = sqlite3_step(stmt);
while (rc == SQLITE_ROW) {
int id = sqlite3_column_int(stmt, 0);
const unsigned char *name = sqlite3_column_text(stmt, 1);
double price = sqlite3_column_double(stmt, 2);
printf("id = %d, name = %s, price = %.2f\n", id, name, price);
rc = sqlite3_step(stmt);
}
sqlite3_finalize(stmt);
sqlite3_close(db);
return 0;
}
```
阅读全文
相关推荐
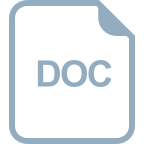
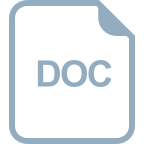
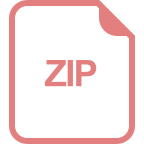
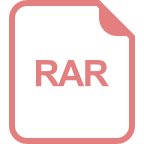
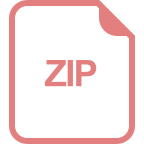
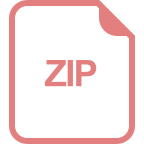
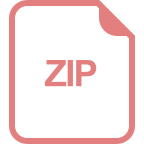
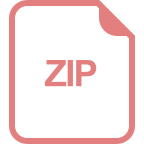
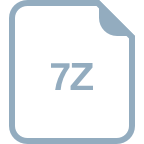
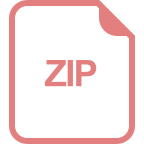
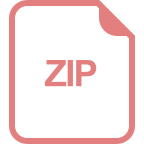
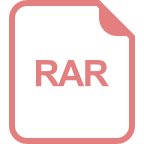
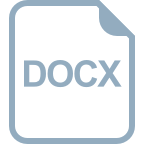
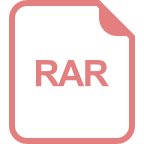
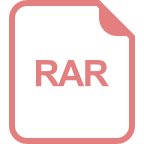
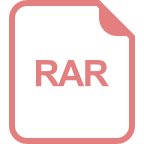
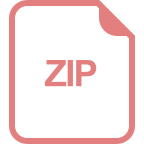
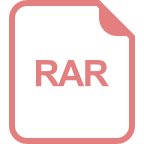