1.题目要求 在主函数中定义一个一维字符数组,从键盘输入一串未排序的字符存入数组,在被调函数(delete)中删去一维数组中所有相同的字符,使之只保留一个,被调函数返回删除的字符个数。主调函数输出删除后的字符串。 例如: 原数组:aabcaacb1212xyz, 删除后:abc12xyz。
时间: 2024-03-09 22:48:06 浏览: 14
好的,这是一道关于字符数组的题目,需要涉及到字符的输入、输出和删除,下面是代码实现:
```c
#include <stdio.h>
#include <string.h>
int delete(char s[]) {
int len = strlen(s);
int count = 0;
for (int i = 0; i < len; i++) {
for (int j = i + 1; j < len; ) {
if (s[i] == s[j]) {
count++;
for (int k = j; k < len - 1; k++) {
s[k] = s[k+1];
}
len--;
} else {
j++;
}
}
}
return count;
}
int main() {
char s[100];
printf("Please input a string: ");
scanf("%s", s);
int count = delete(s);
printf("After delete, the string is: %s\n", s);
printf("The number of deleted characters is: %d\n", count);
return 0;
}
```
代码中的delete函数用于删除字符串中所有相同的字符,保留一个,并返回删除的字符个数。在主函数中,先输入一个字符串,然后调用delete函数进行删除,并输出删除后的字符串和删除的字符个数。
注意,代码中使用了三个循环嵌套,时间复杂度为O(n^3),对于大规模的字符串可能会比较慢,可以考虑使用其他更高效的算法。
相关推荐
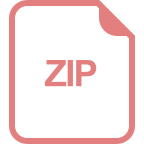














