使用TensorFlow的lstm构建一个回归问题模型
时间: 2023-11-22 10:56:14 浏览: 64
好的,可以的。回归问题通常是指预测连续变量的值,而不是分类问题中的类别。在这里,我们将使用TensorFlow的LSTM模型来预测一个时间序列中下一个值的大小。
首先,我们需要导入必要的库:
```python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
```
然后,我们需要准备数据。在这个例子中,我们将生成一个正弦函数的时间序列,然后使用前几个值来预测下一个值。我们将把时间序列分成训练集和测试集,以便我们可以评估模型的性能。
```python
# 生成正弦函数时间序列
t = np.arange(0, 100, 0.1)
data = np.sin(t)
# 将数据分成训练集和测试集
train_data = data[:800]
test_data = data[800:]
```
接下来,我们需要定义一些超参数,包括模型中LSTM层的数量、每个LSTM层中神经元的数量、批处理大小和迭代次数。
```python
# 定义超参数
num_layers = 2
hidden_size = 64
batch_size = 32
num_steps = 10
num_epochs = 100
```
在这里,我们使用两个LSTM层,每个层有64个神经元。我们还定义了批处理大小为32,这意味着我们将一次性处理32个时间序列。我们还定义了num_steps,这是我们将用来训练模型的时间步数。
接下来,我们需要准备我们的数据。我们将使用前num_steps个值来预测下一个值。我们还将创建一个函数来批处理我们的数据。
```python
# 准备数据
def create_batches(data, batch_size, num_steps):
num_batches = int(len(data)/(batch_size*num_steps))
data = data[:num_batches*batch_size*num_steps]
data = data.reshape((batch_size, -1))
batches = []
for i in range(0, data.shape[1], num_steps):
x = data[:, i:i+num_steps]
y = np.zeros_like(x)
y[:, :-1] = x[:, 1:]
y[:, -1] = data[:, i+num_steps]
batches.append((x, y))
return batches
train_batches = create_batches(train_data, batch_size, num_steps)
```
现在我们已经准备好了数据,我们可以开始构建模型了。我们将使用TensorFlow的LSTMCell和MultiRNNCell函数来定义LSTM层。然后,我们将使用tf.nn.dynamic_rnn函数来构建我们的模型。
```python
# 定义模型
input_data = tf.placeholder(tf.float32, [batch_size, num_steps])
target_data = tf.placeholder(tf.float32, [batch_size, num_steps])
lstm_cells = [tf.contrib.rnn.LSTMCell(hidden_size) for _ in range(num_layers)]
cell = tf.contrib.rnn.MultiRNNCell(lstm_cells)
initial_state = cell.zero_state(batch_size, tf.float32)
with tf.variable_scope('lstm'):
outputs, final_state = tf.nn.dynamic_rnn(cell, tf.expand_dims(input_data, -1), initial_state=initial_state)
output = tf.reshape(outputs, [-1, hidden_size])
target = tf.reshape(target_data, [-1])
weights = tf.Variable(tf.truncated_normal([hidden_size, 1]))
bias = tf.Variable(tf.zeros([1]))
prediction = tf.matmul(output, weights) + bias
loss = tf.reduce_mean(tf.square(prediction - target))
optimizer = tf.train.AdamOptimizer().minimize(loss)
```
在这里,我们定义了两个占位符,一个用于输入数据,另一个用于目标数据。我们还创建了LSTM层,并使用tf.nn.dynamic_rnn函数将其连接起来。我们将输出展平为二维张量,并使用全连接层将其映射到一个单独的输出值。我们使用均方误差作为损失函数,并使用Adam优化器来最小化损失。
现在我们已经定义了模型,我们可以开始训练它了。
```python
# 训练模型
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
for epoch in range(num_epochs):
epoch_loss = 0
for batch in train_batches:
x, y = batch
feed_dict = {input_data: x, target_data: y}
_, loss_val = sess.run([optimizer, loss], feed_dict=feed_dict)
epoch_loss += loss_val
print('Epoch', epoch, 'loss', epoch_loss)
# 在测试集上评估模型
test_batches = create_batches(test_data, batch_size, num_steps)
predictions = []
for batch in test_batches:
x, y = batch
feed_dict = {input_data: x, target_data: y}
prediction_val = sess.run(prediction, feed_dict=feed_dict)
predictions.append(prediction_val)
predictions = np.concatenate(predictions)
plt.plot(test_data[num_steps:], label='True Data')
plt.plot(predictions, label='Predictions')
plt.legend()
plt.show()
```
在这里,我们使用tf.Session来运行我们的模型。我们使用global_variables_initializer函数来初始化模型中的所有变量。然后,我们迭代训练数据,并在每个epoch结束时输出当前损失。最后,我们在测试集上评估模型,并将其预测结果可视化。
这就是使用TensorFlow的LSTM模型来解决回归问题的例子。
相关推荐
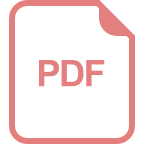
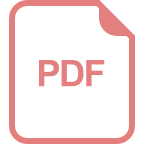
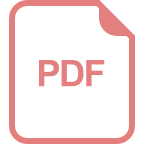














