python 获取oracle表字段以及长度
时间: 2023-08-24 18:14:36 浏览: 283
你可以使用 Python 中的 cx_Oracle 库来获取 Oracle 表的字段以及长度。以下是一个简单的示例代码:
```python
import cx_Oracle
# 连接数据库
conn = cx_Oracle.connect('username/password@hostname:port/sid')
# 创建游标
cur = conn.cursor()
# 查询表结构
cur.execute("SELECT COLUMN_NAME, DATA_TYPE, DATA_LENGTH FROM USER_TAB_COLUMNS WHERE TABLE_NAME = 'your_table_name'")
# 遍历结果集
for col_name, data_type, data_length in cur:
print(col_name, data_type, data_length)
# 关闭游标和连接
cur.close()
conn.close()
```
在代码中,通过连接数据库并创建游标,然后执行 SQL 语句查询指定表的列名、数据类型和数据长度,并遍历结果集打印出来。你可以将 `your_table_name` 替换成你需要查询的表名。
相关问题
PYTHON 读oracle数据库
要在Python中读取Oracle数据库,需要使用Oracle官方提供的Python驱动程序cx_Oracle。以下是一些基本的代码示例:
1. 安装cx_Oracle
```python
pip install cx_Oracle
```
2. 连接数据库
```python
import cx_Oracle
# 连接信息
username = 'your_username'
password = 'your_password'
dsn = cx_Oracle.makedsn('your_host', 'your_port', service_name='your_service_name')
# 连接数据库
conn = cx_Oracle.connect(username, password, dsn)
# 获取游标
cursor = conn.cursor()
```
3. 执行SQL语句
```python
# 执行SQL语句
sql = 'SELECT * FROM your_table'
cursor.execute(sql)
# 获取结果集
result_set = cursor.fetchall()
# 处理结果集
for row in result_set:
print(row)
```
4. 关闭连接
```python
# 关闭游标
cursor.close()
# 关闭连接
conn.close()
```
注意,要在Python代码中使用Oracle数据库,需要在本地安装Oracle客户端或者使用Instant Client。具体安装方法可以参考Oracle官方文档。
阅读全文
相关推荐
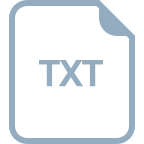
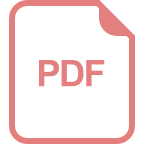
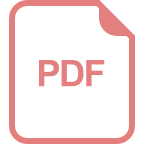
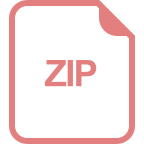
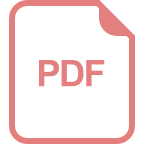
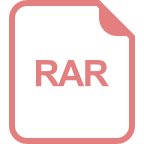
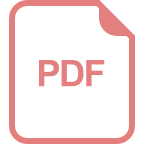
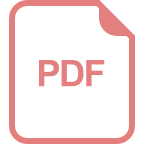
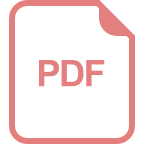
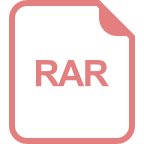
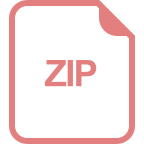
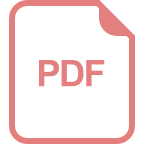