用 QLabel/QImage/QPixmap 来显示图片的示例项目 viewer-demo,实现图片的依次浏览,此时按下 space/ backspace 键,或者用鼠标点击 next/previous 菜单项,可以切换显示下一张/上一张图片;当浏览到最后/第 一张图片时,程序给出相应的提示
时间: 2023-12-16 07:06:23 浏览: 99
好的,我了解了您的问题。这是一个基于 PyQt5 的示例项目,用于演示如何使用 QLabel/QImage/QPixmap 来显示图片,并实现图片的依次浏览。
首先,我们需要创建一个窗口,并在窗口中添加一个 QLabel 来显示图片。然后,我们需要加载图片并将其显示在 QLabel 中。代码如下:
```python
import os
from PyQt5.QtCore import Qt
from PyQt5.QtGui import QPixmap, QImage
from PyQt5.QtWidgets import QMainWindow, QLabel, QAction, QFileDialog
class ImageViewer(QMainWindow):
def __init__(self):
super().__init__()
self.current_image_path = None
self.current_index = 0
self.image_paths = []
# Create QLabel
self.image_label = QLabel(self)
self.image_label.setAlignment(Qt.AlignCenter)
self.setCentralWidget(self.image_label)
# Create actions
self.next_action = QAction("Next", self)
self.next_action.setShortcut("Right")
self.next_action.triggered.connect(self.next_image)
self.previous_action = QAction("Previous", self)
self.previous_action.setShortcut("Left")
self.previous_action.triggered.connect(self.previous_image)
self.open_action = QAction("Open", self)
self.open_action.setShortcut("Ctrl+O")
self.open_action.triggered.connect(self.open_image)
# Create menubar
menubar = self.menuBar()
file_menu = menubar.addMenu("File")
file_menu.addAction(self.open_action)
view_menu = menubar.addMenu("View")
view_menu.addAction(self.previous_action)
view_menu.addAction(self.next_action)
# Set window properties
self.setWindowTitle("Image Viewer")
self.setGeometry(100, 100, 800, 600)
def open_image(self):
file_path, _ = QFileDialog.getOpenFileName(self, "Open Image", "", "Images (*.png *.xpm *.jpg *.bmp)")
if file_path:
self.current_index = 0
self.current_image_path = file_path
self.image_paths = [file_path]
self.load_image(file_path)
def load_image(self, file_path):
image = QImage(file_path)
if image.isNull():
return
pixmap = QPixmap.fromImage(image)
self.image_label.setPixmap(pixmap)
self.setWindowTitle(f"Image Viewer - {os.path.basename(file_path)}")
def next_image(self):
if self.current_index == len(self.image_paths) - 1:
self.statusBar().showMessage("Last image reached!")
return
self.current_index += 1
self.current_image_path = self.image_paths[self.current_index]
self.load_image(self.current_image_path)
def previous_image(self):
if self.current_index == 0:
self.statusBar().showMessage("First image reached!")
return
self.current_index -= 1
self.current_image_path = self.image_paths[self.current_index]
self.load_image(self.current_image_path)
```
在这个示例项目中,我们定义了一个 ImageViewer 类,这个类继承了 QMainWindow 类。我们在这个类的构造函数中创建了一个 QLabel,并将其设置为窗口的中央小部件。
我们还定义了三个 QAction:next_action、previous_action 和 open_action。这些 QAction 可以通过菜单栏或者快捷键来触发。
在 open_action 的触发函数中,我们使用 QFileDialog 来打开一个文件对话框,让用户选择要打开的图片文件。如果用户选择了一个文件,我们就将这个文件的路径加入到 image_paths 列表中,并调用 load_image() 函数来加载并显示这个图片。
在 load_image() 函数中,我们使用 QImage 来加载图片,并将其转换为 QPixmap。然后,我们将 QPixmap 设置为 QLabel 的 pixmap,以便将其显示在窗口中。
在 next_image() 和 previous_image() 函数中,我们使用 current_index 来跟踪当前显示的图片在 image_paths 列表中的索引。当用户按下 "Next" 或 "Previous" 按钮时,我们更新 current_index,并调用 load_image() 函数来加载并显示下一张/上一张图片。如果用户已经浏览到了最后一张/第一张图片,我们将在状态栏中显示相应的提示信息。
最后,我们需要创建一个应用程序对象,并在其上运行 ImageViewer 窗口:
```python
import sys
from PyQt5.QtWidgets import QApplication
if __name__ == '__main__':
app = QApplication(sys.argv)
viewer = ImageViewer()
viewer.show()
sys.exit(app.exec_())
```
这样,我们就完成了基于 PyQt5 的图片浏览器示例项目。
阅读全文
相关推荐
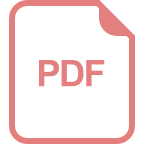
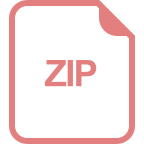



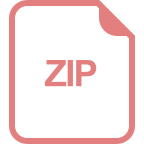










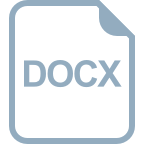
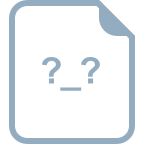