java 最全的post方法
时间: 2024-02-16 11:53:17 浏览: 152
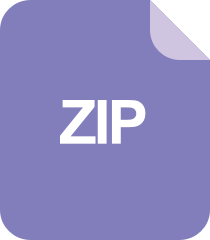
java发送http/https请求(get/post)Demo,亲测可用
以下是 Java 中最常用的 POST 方法:
1. 使用 HttpURLConnection 发送 POST 请求:
```java
try {
// 创建 URL 对象
URL url = new URL("http://www.example.com/api");
// 创建 HttpURLConnection 对象
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
// 设置请求方法为 POST
conn.setRequestMethod("POST");
// 允许向服务器输出内容
conn.setDoOutput(true);
// 设置请求参数,多个参数使用 & 分隔
String params = "param1=value1¶m2=value2";
OutputStream os = conn.getOutputStream();
os.write(params.getBytes());
os.flush();
os.close();
// 获取服务器返回的数据
InputStream is = conn.getInputStream();
BufferedReader br = new BufferedReader(new InputStreamReader(is));
String line;
StringBuilder result = new StringBuilder();
while ((line = br.readLine()) != null) {
result.append(line);
}
br.close();
is.close();
// 打印结果
System.out.println(result.toString());
} catch (Exception e) {
e.printStackTrace();
}
```
2. 使用 HttpClient 发送 POST 请求:
```java
try {
// 创建 CloseableHttpClient 对象
CloseableHttpClient httpclient = HttpClients.createDefault();
// 创建 HttpPost 对象
HttpPost httppost = new HttpPost("http://www.example.com/api");
// 设置请求参数
List<NameValuePair> params = new ArrayList<NameValuePair>();
params.add(new BasicNameValuePair("param1", "value1"));
params.add(new BasicNameValuePair("param2", "value2"));
httppost.setEntity(new UrlEncodedFormEntity(params, "UTF-8"));
// 执行请求并获取服务器返回的数据
CloseableHttpResponse response = httpclient.execute(httppost);
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity, "UTF-8");
// 打印结果
System.out.println(result);
// 关闭连接
response.close();
httpclient.close();
} catch (Exception e) {
e.printStackTrace();
}
```
以上两种方法都可以用来发送 POST 请求,具体使用哪种方法可以根据具体情况选择。
阅读全文
相关推荐
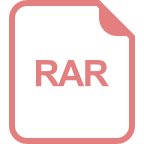
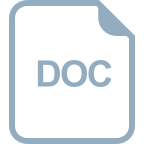
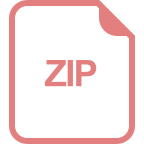
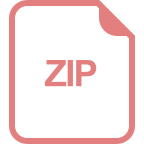
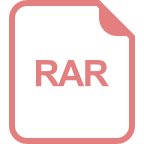
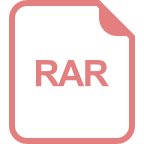
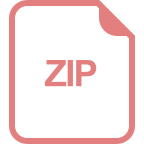
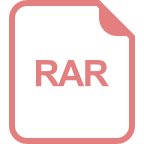
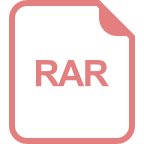
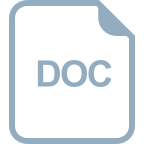
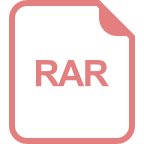
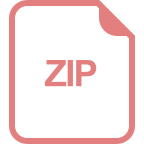
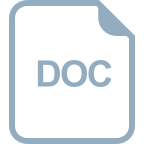
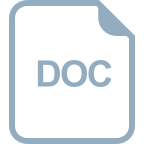
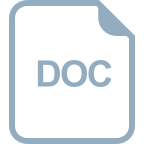
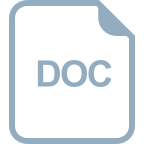
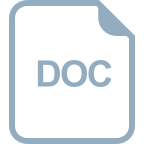
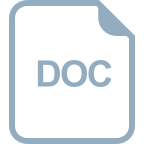