python将字符串转化为datatime
时间: 2023-12-31 08:03:50 浏览: 137
Sure, I can assist you with that. Here's an example code to convert a string to datetime format in Python:
```
from datetime import datetime
string_date = '2021-09-15 16:30:00'
datetime_obj = datetime.strptime(string_date, '%Y-%m-%d %H:%M:%S')
```
In the above code, the `strptime` method is used to parse the string date, and the `%Y-%m-%d %H:%M:%S` format code is used to represent the string format. You can replace the `string_date` variable with the actual string date that you want to convert. Let me know if you have any further questions.
相关问题
python 中如何把字符串转换成datatime
在 Python 中,可以使用 `datetime` 模块中的 `datetime.strptime()` 函数将字符串转换成 `datetime` 类型,代码示例如下:
```python
from datetime import datetime
date_string = "2021-08-25 10:30:00"
date_format = "%Y-%m-%d %H:%M:%S"
datetime_obj = datetime.strptime(date_string, date_format)
print(datetime_obj)
```
输出:
```
2021-08-25 10:30:00
```
在上面的代码中,`date_string` 是要转换的字符串,`date_format` 是字符串的格式,`datetime_obj` 是转换后的 `datetime` 对象。`%Y` 表示年份,`%m` 表示月份,`%d` 表示日期,`%H` 表示小时,`%M` 表示分钟,`%S` 表示秒。你可以根据实际情况修改 `date_string` 和 `date_format` 的值。
字符串如何转化为datatime
在Python中,可以使用datetime模块将字符串转换为datetime对象。具体的方法为使用datetime.datetime.strptime()函数,该函数可以将指定格式的字符串转换为datetime对象。
例如,假设有一个字符串表示为"2021-10-01 12:00:00",并且想要将其转换为datetime对象,可以使用以下代码:
```python
import datetime
date_str = "2021-10-01 12:00:00"
date_format = "%Y-%m-%d %H:%M:%S"
datetime_obj = datetime.datetime.strptime(date_str, date_format)
print(datetime_obj)
```
其中,date_str表示要转换的字符串,date_format表示字符串的格式,"%Y-%m-%d %H:%M:%S"表示年-月-日 时:分:秒的格式。最后的datetime_obj即为转换后得到的datetime对象。
阅读全文
相关推荐
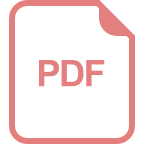







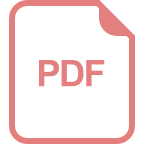
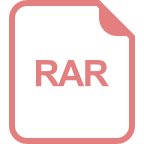





