帮我写一个php购物车网页设计代码
时间: 2023-08-28 22:19:54 浏览: 128
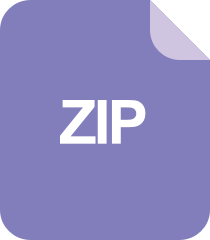
基于PHP实现的金星session购物车实例_cart_电子商务(源代码+html+毕业设计).zip
好的,以下是一个简单的 PHP 购物车网页设计代码:
```
<?php
// 连接数据库
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "myDB";
$conn = new mysqli($servername, $username, $password, $dbname);
// 检查连接是否成功
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// 检索商品信息
$sql = "SELECT id, name, price, image FROM products";
$result = $conn->query($sql);
// 开始 HTML 输出
echo "<table>";
echo "<tr><th>Name</th><th>Price</th><th>Image</th><th>Action</th></tr>";
// 输出商品信息
while($row = $result->fetch_assoc()) {
echo "<tr>";
echo "<td>" . $row["name"] . "</td>";
echo "<td>" . $row["price"] . "</td>";
echo "<td><img src='" . $row["image"] . "'></td>";
echo "<td><button onclick='addToCart(" . $row["id"] . ")'>Add to Cart</button></td>";
echo "</tr>";
}
echo "</table>";
// 关闭数据库连接
$conn->close();
?>
<script>
// 添加商品到购物车
function addToCart(id) {
var cart = JSON.parse(getCookie("cart") || "[]");
var item = cart.find(function(item) { return item.id === id });
if (item) {
item.quantity += 1;
} else {
cart.push({ id: id, quantity: 1 });
}
setCookie("cart", JSON.stringify(cart), 365);
}
// 获取 Cookie 值
function getCookie(name) {
var value = "; " + document.cookie;
var parts = value.split("; " + name + "=");
if (parts.length == 2) return parts.pop().split(";").shift();
}
// 设置 Cookie 值
function setCookie(name, value, days) {
var expires = "";
if (days) {
var date = new Date();
date.setTime(date.getTime() + (days * 24 * 60 * 60 * 1000));
expires = "; expires=" + date.toUTCString();
}
document.cookie = name + "=" + (value || "") + expires + "; path=/";
}
</script>
<?php
// 检查购物车信息并计算总价
$cart = json_decode($_COOKIE["cart"] ?? "[]", true);
$total = 0;
foreach ($cart as $item) {
$sql = "SELECT price FROM products WHERE id = " . $item["id"];
$result = $conn->query($sql);
if ($result->num_rows > 0) {
$row = $result->fetch_assoc();
$total += $row["price"] * $item["quantity"];
}
}
// 输出购物车信息
echo "<p>Items in cart: " . count($cart) . "</p>";
echo "<p>Total: $" . $total . "</p>";
?>
<button onclick="location.href='checkout.php'">Checkout</button>
```
这个代码包括了连接数据库、检索商品信息、输出商品列表、添加商品到购物车、获取 Cookie、设置 Cookie、计算总价、输出购物车信息和结账按钮等功能。你可以根据你的具体需求进行修改和定制。
阅读全文
相关推荐
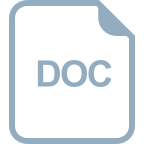
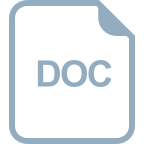
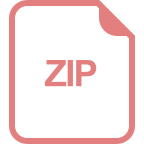
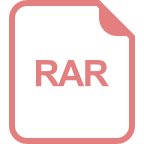
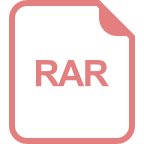
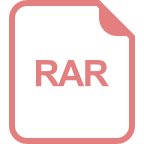
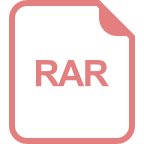
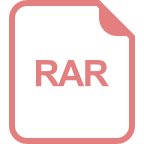
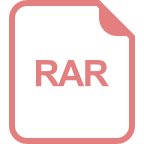
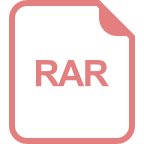
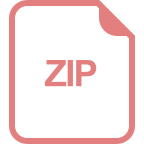
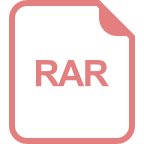
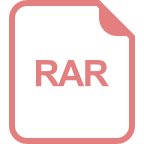
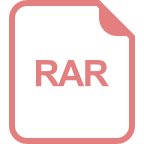
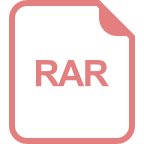
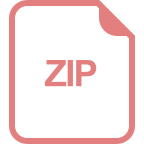
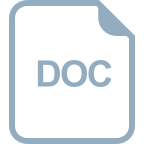
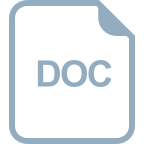