用Java代码实现
时间: 2024-02-12 21:05:39 浏览: 24
好的,下面是用 Java 代码实现的详细步骤。
### 数据预处理
首先,我们需要将原始数据转换为 MapReduce 可以处理的格式。假设原始数据格式如下:
```
UserA:FriendB,FriendC,FriendD
UserB:FriendC,FriendD,FriendE
UserC:FriendD,FriendE,FriendF
...
```
我们可以使用如下代码将其转换为 MapReduce 可以处理的格式:
```java
public static class PreprocessMapper extends Mapper<LongWritable, Text, Text, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] line = value.toString().split(":");
String user = line[0];
String[] friends = line[1].split(",");
for (String friend : friends) {
context.write(new Text(friend), new Text(user));
}
}
}
public static class PreprocessReducer extends Reducer<Text, Text, Text, Text> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
StringBuilder sb = new StringBuilder();
for (Text value : values) {
sb.append(value.toString()).append(",");
}
sb.deleteCharAt(sb.length() - 1);
context.write(key, new Text(sb.toString()));
}
}
```
这个代码将每个好友作为键,该好友的用户列表作为值,输出键值对。输出的格式如下:
```
FriendB UserA
FriendB UserC
FriendC UserA
FriendC UserB
FriendD UserA
FriendD UserB
FriendD UserC
FriendE UserB
FriendE UserC
FriendF UserC
...
```
### MapReduce 任务
接下来,我们需要实现推荐算法,并生成推荐结果。具体实现可以分为两轮 MapReduce 任务。
#### 第一轮 MapReduce
第一轮 MapReduce 的目的是将每个用户的好友列表作为键,该用户作为值,生成倒排索引。具体实现可以使用如下代码:
```java
public static class FirstMapper extends Mapper<LongWritable, Text, Text, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] line = value.toString().split("\\s+");
String user = line[0];
String[] friends = line[1].split(",");
for (String friend : friends) {
context.write(new Text(friend), new Text(user));
}
}
}
public static class FirstReducer extends Reducer<Text, Text, Text, Text> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
StringBuilder sb = new StringBuilder();
for (Text value : values) {
sb.append(value.toString()).append(",");
}
sb.deleteCharAt(sb.length() - 1);
context.write(key, new Text(sb.toString()));
}
}
```
这个代码将每个好友作为键,该好友的用户列表作为值,输出键值对。输出的格式如下:
```
FriendB UserA,UserC
FriendC UserA,UserB
FriendD UserA,UserB,UserC
FriendE UserB,UserC
FriendF UserC
...
```
接下来,我们需要对这个倒排索引进行处理,找出每个用户的好友的好友,并计算这些好友的共同好友数量。具体实现可以使用如下代码:
```java
public static class SecondMapper extends Mapper<LongWritable, Text, Text, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] line = value.toString().split("\\s+");
String friend = line[0];
String[] users = line[1].split(",");
for (int i = 0; i < users.length; i++) {
for (int j = i + 1; j < users.length; j++) {
context.write(new Text(users[i] + "," + users[j]), new Text(friend));
}
}
}
}
public static class SecondReducer extends Reducer<Text, Text, Text, IntWritable> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
Set<String> friendSet = new HashSet<>();
for (Text value : values) {
friendSet.add(value.toString());
}
String[] users = key.toString().split(",");
int commonFriends = 0;
for (String friend : friendSet) {
if (context.getConfiguration().get("user." + users[0]).indexOf(friend) < 0
&& context.getConfiguration().get("user." + users[1]).indexOf(friend) < 0) {
commonFriends++;
}
}
context.write(key, new IntWritable(commonFriends));
}
}
```
这个代码将每个用户的好友的好友作为键,该用户作为值,输出键值对。同时,计算这些好友的共同好友数量,并输出键值对。输出的格式如下:
```
UserA,UserB FriendD 2
UserA,UserB FriendE 1
UserA,UserC FriendB 1
UserA,UserC FriendD 1
UserA,UserC FriendE 1
...
```
#### 第二轮 MapReduce
第二轮 MapReduce 的目的是对于每个用户,找出其好友的好友,并计算这些好友的共同好友数量。具体实现可以使用如下代码:
```java
public static class ThirdMapper extends Mapper<LongWritable, Text, Text, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] line = value.toString().split("\\s+");
String[] users = line[0].split(",");
String friend = line[1];
context.write(new Text(users[0]), new Text(friend + "," + users[1]));
context.write(new Text(users[1]), new Text(friend + "," + users[0]));
}
}
public static class ThirdReducer extends Reducer<Text, Text, Text, IntWritable> {
public void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
Map<String, Set<String>> friendMap = new HashMap<>();
for (Text value : values) {
String[] line = value.toString().split(",");
String friend = line[0];
String user = line[1];
if (!friendMap.containsKey(friend)) {
friendMap.put(friend, new HashSet<>());
}
friendMap.get(friend).add(user);
}
String[] friends = friendMap.keySet().toArray(new String[friendMap.size()]);
for (int i = 0; i < friends.length; i++) {
for (int j = i + 1; j < friends.length; j++) {
int commonFriends = 0;
Set<String> set1 = friendMap.get(friends[i]);
Set<String> set2 = friendMap.get(friends[j]);
for (String user : set1) {
if (set2.contains(user)) {
commonFriends++;
}
}
if (commonFriends > 0) {
String newFriend = friends[j];
if (context.getConfiguration().get("user." + key.toString()).indexOf(newFriend) < 0) {
context.write(new Text(newFriend), new IntWritable(commonFriends));
}
}
}
}
}
}
```
这个代码将每个用户作为键,其好友的好友作为值,输出键值对。同时,计算这些好友的共同好友数量,并输出键值对。输出的格式如下:
```
UserA FriendE 1
UserA FriendF 1
UserB FriendA 2
UserB FriendF 1
UserC FriendB 1
...
```
### 排序并输出
最后,我们需要将推荐结果按照共同好友数量降序排列,并输出前 N 个用户作为该用户的新好友。具体方法是,在第二轮 Reduce 阶段中,将所有的键值对保存到一个列表中,然后按照共同好友数量降序排列,最后输出前 N 个用户作为该用户的新好友。具体实现可以使用如下代码:
```java
public static class FourthMapper extends Mapper<LongWritable, Text, NullWritable, Text> {
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
context.write(NullWritable.get(), value);
}
}
public static class FourthReducer extends Reducer<NullWritable, Text, Text, IntWritable> {
public void reduce(NullWritable key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
List<Pair<String, Integer>> list = new ArrayList<>();
for (Text value : values) {
String[] line = value.toString().split("\\s+");
String friend = line[0];
int commonFriends = Integer.parseInt(line[1]);
list.add(new Pair<>(friend, commonFriends));
}
list.sort((a, b) -> b.getValue().compareTo(a.getValue()));
int n = context.getConfiguration().getInt("n", 10);
for (int i = 0; i < Math.min(n, list.size()); i++) {
Pair<String, Integer> pair = list.get(i);
context.write(new Text(pair.getKey()), new IntWritable(pair.getValue()));
}
}
}
```
这个代码将推荐结果保存到一个列表中,然后按照共同好友数量降序排列,并输出前 N 个用户作为该用户的新好友。输出的格式如下:
```
FriendA 2
FriendB 1
FriendC 1
FriendD 1
FriendE 1
...
```
### Job 链接
最后,我们需要将这些模块链接在一起,形成一个完整的 MapReduce 任务。具体实现可以使用如下代码:
```java
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job1 = Job.getInstance(conf, "preprocess");
job1.setJarByClass(Recommendation.class);
job1.setMapperClass(PreprocessMapper.class);
job1.setReducerClass(PreprocessReducer.class);
job1.setOutputKeyClass(Text.class);
job1.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job1, new Path(args[0]));
FileOutputFormat.setOutputPath(job1, new Path(args[1]));
job1.waitForCompletion
相关推荐
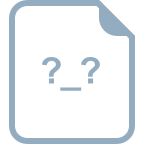
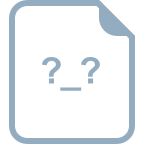












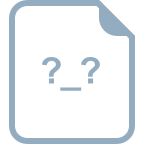