ARIMA完整python代码
时间: 2023-07-02 09:09:25 浏览: 53
ARIMA是一种时间序列预测模型,下面是一个完整的Python代码实现:
首先,我们需要导入必要的库并加载数据集:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from statsmodels.tsa.arima_model import ARIMA
# 加载数据集
df = pd.read_csv('data.csv', parse_dates=['date'], index_col='date')
```
接着,我们需要对时间序列进行可视化分析,以便了解其趋势、季节性和周期性:
```python
# 可视化时间序列
plt.figure(figsize=(12, 8))
plt.plot(df)
plt.xlabel('Year')
plt.ylabel('Sales')
plt.show()
```
然后,我们可以使用差分运算来平稳时间序列,以便更好地使用ARIMA模型:
```python
# 平稳时间序列
diff = df.diff().dropna()
# 可视化平稳时间序列
plt.figure(figsize=(12, 8))
plt.plot(diff)
plt.xlabel('Year')
plt.ylabel('Sales')
plt.show()
```
接下来,我们可以使用自相关和部分自相关图来确定ARIMA模型的参数:
```python
# 自相关和部分自相关图
from statsmodels.graphics.tsaplots import plot_acf, plot_pacf
plot_acf(diff)
plt.show()
plot_pacf(diff)
plt.show()
```
根据自相关和部分自相关图,我们可以确定ARIMA模型的参数。在这个例子中,我们选择了ARIMA(2,1,1)模型:
```python
# ARIMA模型
model = ARIMA(df, order=(2,1,1))
model_fit = model.fit(disp=0)
print(model_fit.summary())
```
最后,我们可以使用ARIMA模型进行预测:
```python
# 预测未来n期
n = 10
forecast = model_fit.forecast(steps=n)[0]
# 可视化预测结果
plt.figure(figsize=(12, 8))
plt.plot(df)
plt.plot(pd.date_range(start=df.index[-1], periods=n+1, freq='Y')[1:], forecast, color='r')
plt.xlabel('Year')
plt.ylabel('Sales')
plt.show()
```
这就是一个完整的ARIMA模型的Python代码实现。
相关推荐
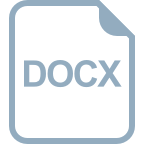
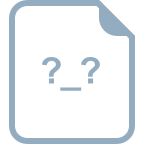
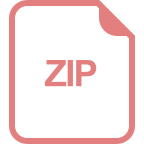
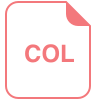
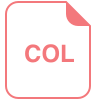






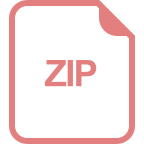
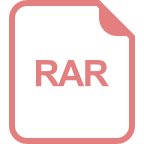
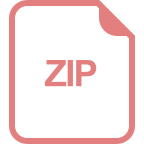