用python将正序序列和逆序序列都利用 WordEmbedding技术生成词向量,分别作为本文设计的Attention-BasedLSTM文本分类模型的输入序列
时间: 2024-05-05 11:18:04 浏览: 14
好的,您的问题是如何使用 Python 生成正序序列和逆序序列的词向量,并作为 Attention-Based LSTM 文本分类模型的输入序列。
首先,我们需要导入所需的库:
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.preprocessing.sequence import pad_sequences
from tensorflow.keras.layers import Input, Embedding, LSTM, Dense, Dropout, Attention
from tensorflow.keras.models import Model
```
接下来,我们需要加载训练好的词向量模型,这里以 GloVe 为例:
```python
EMBEDDING_DIM = 100
MAX_SEQ_LEN = 100
MAX_NUM_WORDS = 20000
# load pre-trained word embeddings
words_list = []
with open('glove.6B.100d.txt', encoding='utf-8') as f:
for line in f:
values = line.split()
word = values[0]
words_list.append(word)
# create word-to-index dictionary
word_index = {}
for idx, word in enumerate(words_list):
word_index[word] = idx + 1
# create embedding matrix
embedding_matrix = np.zeros((MAX_NUM_WORDS + 1, EMBEDDING_DIM))
with open('glove.6B.100d.txt', encoding='utf-8') as f:
for line in f:
values = line.split()
word = values[0]
if word in word_index:
idx = word_index[word]
embedding_matrix[idx] = np.asarray(values[1:], dtype=np.float32)
```
然后,我们可以定义模型的输入和输出:
```python
input_seqs = Input(shape=(MAX_SEQ_LEN,), dtype='int32')
embedding_layer = Embedding(
MAX_NUM_WORDS + 1,
EMBEDDING_DIM,
weights=[embedding_matrix],
input_length=MAX_SEQ_LEN,
trainable=False
)(input_seqs)
lstm_layer = LSTM(64, return_sequences=True)(embedding_layer)
attention_layer = Attention()([lstm_layer, lstm_layer])
dense_layer = Dense(64, activation='relu')(attention_layer)
dropout_layer = Dropout(0.5)(dense_layer)
output_layer = Dense(1, activation='sigmoid')(dropout_layer)
model = Model(inputs=input_seqs, outputs=output_layer)
```
接着,我们定义模型的损失函数和优化器:
```python
model.compile(
loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy']
)
```
最后,我们可以使用 pad_sequences 函数将正序序列和逆序序列转换为定长序列,并输入模型中进行训练:
```python
## assume X_train_pos and X_train_neg are the positive and negative training data, respectively
X_train_pos_seq = pad_sequences(X_train_pos, maxlen=MAX_SEQ_LEN)
X_train_neg_seq = pad_sequences(X_train_neg, maxlen=MAX_SEQ_LEN)
## concatenate positive and negative sequences and labels
X_train_seq = np.concatenate((X_train_pos_seq, X_train_neg_seq))
y_train = np.concatenate((np.ones(len(X_train_pos_seq)), np.zeros(len(X_train_neg_seq))))
## shuffle the training data
np.random.seed(7)
shuffle_indices = np.random.permutation(np.arange(len(X_train_seq)))
X_train_seq = X_train_seq[shuffle_indices]
y_train = y_train[shuffle_indices]
## train the model
model.fit(X_train_seq, y_train, validation_split=0.1, epochs=10, batch_size=128)
```
希望以上内容对您有所帮助。如果您有其他问题,请随时提出。
相关推荐
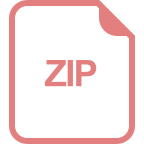
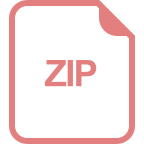














