c语言链栈的基本操作,代码展示
时间: 2023-10-17 20:11:24 浏览: 46
下面是C语言链栈的基本操作代码展示:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链栈结构体
struct node {
int data;
struct node *next;
};
// 初始化链栈
void initStack(struct node **top) {
*top = NULL;
}
// 判断链栈是否为空
int isEmpty(struct node *top) {
return top == NULL;
}
// 入栈操作
void push(struct node **top, int data) {
struct node *newNode = (struct node*)malloc(sizeof(struct node));
if (newNode == NULL) {
printf("内存分配失败");
exit(1);
}
newNode->data = data;
newNode->next = *top;
*top = newNode;
}
// 出栈操作
int pop(struct node **top) {
if (isEmpty(*top)) {
printf("链栈为空");
exit(1);
}
int data = (*top)->data;
struct node *temp = *top;
*top = (*top)->next;
free(temp);
return data;
}
// 获取栈顶元素
int peek(struct node *top) {
if (isEmpty(top)) {
printf("链栈为空");
exit(1);
}
return top->data;
}
// 输出链栈
void display(struct node *top) {
if (isEmpty(top)) {
printf("链栈为空");
return;
}
struct node *temp = top;
while (temp != NULL) {
printf("%d->", temp->data);
temp = temp->next;
}
printf("NULL");
}
int main() {
struct node *top;
initStack(&top);
push(&top, 1);
push(&top, 2);
push(&top, 3);
printf("链栈元素为:");
display(top);
printf("\n");
printf("栈顶元素为:%d\n", peek(top));
printf("出栈元素为:%d\n", pop(&top));
printf("链栈元素为:");
display(top);
printf("\n");
return 0;
}
```
注:上述代码中,`struct node` 表示链栈的节点,包含 `data` 和 `next` 两个成员变量。`top` 表示链栈的栈顶节点。`initStack` 函数用于初始化链栈,将栈顶节点设置为 `NULL`。`isEmpty` 函数用于判断链栈是否为空。`push` 函数用于入栈操作,将新的节点插入链表头部。`pop` 函数用于出栈操作,从链表头部取出节点并删除。`peek` 函数用于获取栈顶元素。`display` 函数用于输出链栈。`main` 函数中展示了链栈的基本操作,包括初始化、入栈、获取栈顶元素、出栈和输出链栈等。
相关推荐
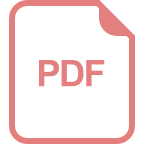












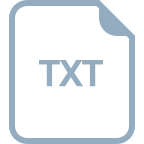
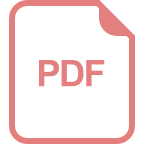