嵌入式超声测距串口模式
时间: 2023-07-30 07:06:51 浏览: 95
嵌入式超声波测距一般会通过串口进行数据的传输。串口模式可以通过嵌入式系统的UART模块实现,具体步骤如下:
1. 配置串口波特率,数据位、停止位、校验位等参数;
2. 实现超声波模块的触发和接收,当触发信号到达时,启动计时器开始计时,等待接收信号返回,当接收到信号后停止计时器,并将计时器的数值通过串口发送出去;
3. 在上位机端,通过串口接收到计时器的数值后,可以根据声速和时间差计算出距离。
需要注意的是,串口通信时需要保证发送方和接收方的波特率、数据位、停止位、校验位等参数一致,否则数据传输可能会出现问题。
相关问题
写一个嵌入式在串口模式下的超声测距
以下是一个基于STM32F103单片机的嵌入式超声波测距程序,通过串口模式进行数据传输。该程序使用PA0作为超声波模块的触发引脚,PA1作为超声波模块的接收引脚,使用USART1作为串口通信模块,串口配置为115200波特率,8位数据位,1位停止位,无校验位。
```
#include "stm32f10x.h"
#include "stdio.h"
#define TRIG_PIN GPIO_Pin_0
#define ECHO_PIN GPIO_Pin_1
#define TRIG_PORT GPIOA
#define ECHO_PORT GPIOA
#define USART USART1
void GPIO_Configuration(void);
void USART_Configuration(void);
void SendData(uint8_t data);
int main(void)
{
GPIO_Configuration();
USART_Configuration();
uint32_t distance;
uint32_t timeout = 1000000;
while(1)
{
// 发送超声波触发信号
GPIO_SetBits(TRIG_PORT, TRIG_PIN);
delay_us(10);
GPIO_ResetBits(TRIG_PORT, TRIG_PIN);
// 等待接收信号
while(GPIO_ReadInputDataBit(ECHO_PORT, ECHO_PIN) == RESET && timeout--);
if(timeout == 0)
{
printf("timeout\r\n");
timeout = 1000000;
continue;
}
// 开始计时
TIM_Cmd(TIM2, ENABLE);
while(GPIO_ReadInputDataBit(ECHO_PORT, ECHO_PIN) == SET && timeout--);
TIM_Cmd(TIM2, DISABLE);
if(timeout == 0)
{
printf("timeout\r\n");
timeout = 1000000;
continue;
}
// 计算距离
distance = TIM_GetCounter(TIM2) * 17;
printf("distance: %d cm\r\n", distance);
timeout = 1000000;
delay_ms(500);
}
}
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = TRIG_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(TRIG_PORT, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = ECHO_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(ECHO_PORT, &GPIO_InitStructure);
}
void USART_Configuration(void)
{
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART, &USART_InitStructure);
USART_Cmd(USART, ENABLE);
}
void SendData(uint8_t data)
{
while(USART_GetFlagStatus(USART, USART_FLAG_TXE) == RESET);
USART_SendData(USART, data);
}
void delay_us(uint32_t us)
{
uint32_t i;
while(us--)
{
i = 10;
while(i--);
}
}
void delay_ms(uint16_t ms)
{
while(ms--)
{
delay_us(1000);
}
}
```
需要注意的是,以上代码只是一个示例程序,具体实现时还需要根据具体超声波模块的接口和信号特性进行相应的调整。
阅读全文
相关推荐
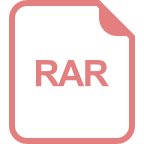
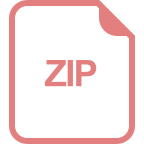
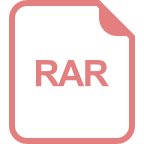
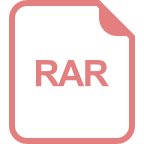
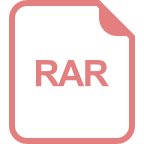
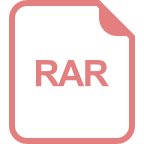
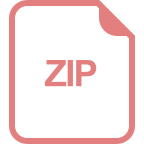
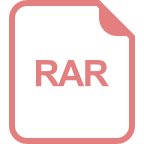
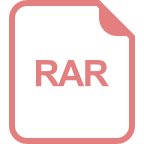
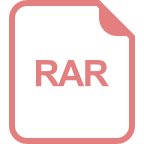
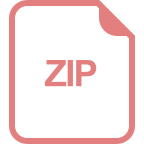
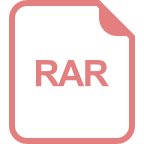
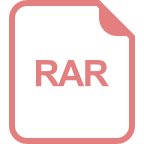
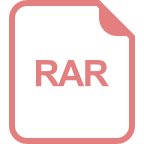
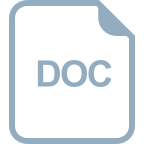