c语言数据结构使用链表的应用编写一个人事管理系统,要输出相应界面:用链式存储方法实现。 每个职工的信息包括:工号、姓名、性别、出生年月、籍贯、部门,能实现输入: 按工号或者姓名或者性别或者部门查找: 插入: 按工号或者姓名或者性别或者部门删除,按工号或者出生年月进行升序或者降序,按工号进行修改职工的信息 ,分别统计30岁以下,30-50和50岁以上,三个年龄段的人数的代码
时间: 2024-02-01 11:13:51 浏览: 33
以下是一个简单的人事管理系统的代码,包括链表的定义和实现,以及各种操作函数的实现。代码仅供参考,可能有不足之处,欢迎指正。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
//定义职工结构体
typedef struct Employee {
int id; //工号
char name[20]; //姓名
char gender[5]; //性别
char birth[20]; //出生年月
char hometown[20]; //籍贯
char department[20]; //部门
struct Employee* next; //指向下一个职工节点的指针
} Employee;
//定义链表结构体
typedef struct EmployeeList {
int count; //职工数量
Employee* head; //指向链表头节点的指针
} EmployeeList;
//初始化链表
void initList(EmployeeList* list) {
list->count = 0;
list->head = NULL;
}
//创建新的职工节点
Employee* createEmployee(int id, const char* name, const char* gender, const char* birth, const char* hometown, const char* department) {
Employee* employee = (Employee*)malloc(sizeof(Employee));
employee->id = id;
strcpy(employee->name, name);
strcpy(employee->gender, gender);
strcpy(employee->birth, birth);
strcpy(employee->hometown, hometown);
strcpy(employee->department, department);
employee->next = NULL;
return employee;
}
//在链表末尾添加新的职工节点
void addEmployee(EmployeeList* list, Employee* employee) {
if (list->head == NULL) {
list->head = employee;
} else {
Employee* current = list->head;
while (current->next != NULL) {
current = current->next;
}
current->next = employee;
}
list->count++;
}
//按工号或者姓名或者性别或者部门查找职工节点
Employee* findEmployee(EmployeeList* list, const char* keyword) {
Employee* current = list->head;
while (current != NULL) {
if (strstr(current->name, keyword) != NULL ||
strstr(current->gender, keyword) != NULL ||
strstr(current->hometown, keyword) != NULL ||
strstr(current->department, keyword) != NULL ||
current->id == atoi(keyword)) {
return current;
}
current = current->next;
}
return NULL;
}
//按工号或者姓名或者性别或者部门删除职工节点
void removeEmployee(EmployeeList* list, const char* keyword) {
Employee* current = list->head;
Employee* prev = NULL;
while (current != NULL) {
if (strstr(current->name, keyword) != NULL ||
strstr(current->gender, keyword) != NULL ||
strstr(current->hometown, keyword) != NULL ||
strstr(current->department, keyword) != NULL ||
current->id == atoi(keyword)) {
if (prev == NULL) {
list->head = current->next;
} else {
prev->next = current->next;
}
free(current);
list->count--;
return;
}
prev = current;
current = current->next;
}
}
//按工号或者出生年月进行升序或者降序排序
void sortEmployee(EmployeeList* list, int ascending) {
Employee* current = list->head;
Employee* prev = NULL;
int swapped = 1;
while (swapped) {
swapped = 0;
while (current != NULL && current->next != NULL) {
if ((ascending && current->id > current->next->id) ||
(!ascending && current->id < current->next->id)) {
Employee* next = current->next;
current->next = next->next;
next->next = current;
if (prev != NULL) {
prev->next = next;
} else {
list->head = next;
}
prev = next;
swapped = 1;
} else {
prev = current;
current = current->next;
}
}
}
}
//按工号修改职工信息
void updateEmployee(EmployeeList* list, int id) {
Employee* current = list->head;
while (current != NULL) {
if (current->id == id) {
printf("请输入新的姓名:");
scanf("%s", current->name);
printf("请输入新的性别:");
scanf("%s", current->gender);
printf("请输入新的出生年月:");
scanf("%s", current->birth);
printf("请输入新的籍贯:");
scanf("%s", current->hometown);
printf("请输入新的部门:");
scanf("%s", current->department);
return;
}
current = current->next;
}
}
//统计30岁以下,30-50和50岁以上,三个年龄段的人数
void countEmployee(EmployeeList* list) {
Employee* current = list->head;
int count1 = 0, count2 = 0, count3 = 0;
while (current != NULL) {
int year = atoi(strtok(current->birth, "-"));
int age = 2021 - year;
if (age < 30) {
count1++;
} else if (age >= 30 && age <= 50) {
count2++;
} else {
count3++;
}
current = current->next;
}
printf("30岁以下的人数:%d\n", count1);
printf("30-50岁的人数:%d\n", count2);
printf("50岁以上的人数:%d\n", count3);
}
//输出菜单
void printMenu() {
printf("人事管理系统\n");
printf("1. 添加职工\n");
printf("2. 查找职工\n");
printf("3. 删除职工\n");
printf("4. 排序职工\n");
printf("5. 修改职工信息\n");
printf("6. 统计职工数量\n");
printf("7. 退出系统\n");
printf("请选择操作:");
}
int main() {
EmployeeList list;
initList(&list);
int choice;
do {
printMenu();
scanf("%d", &choice);
switch (choice) {
case 1: {
int id;
char name[20], gender[5], birth[20], hometown[20], department[20];
printf("请输入工号:");
scanf("%d", &id);
printf("请输入姓名:");
scanf("%s", name);
printf("请输入性别:");
scanf("%s", gender);
printf("请输入出生年月(格式为YYYY-MM-DD):");
scanf("%s", birth);
printf("请输入籍贯:");
scanf("%s", hometown);
printf("请输入部门:");
scanf("%s", department);
Employee* employee = createEmployee(id, name, gender, birth, hometown, department);
addEmployee(&list, employee);
printf("添加职工成功!\n");
break;
}
case 2: {
char keyword[20];
printf("请输入关键字:");
scanf("%s", keyword);
Employee* employee = findEmployee(&list, keyword);
if (employee != NULL) {
printf("工号:%d\n", employee->id);
printf("姓名:%s\n", employee->name);
printf("性别:%s\n", employee->gender);
printf("出生年月:%s\n", employee->birth);
printf("籍贯:%s\n", employee->hometown);
printf("部门:%s\n", employee->department);
} else {
printf("未找到符合条件的职工!\n");
}
break;
}
case 3: {
char keyword[20];
printf("请输入关键字:");
scanf("%s", keyword);
removeEmployee(&list, keyword);
printf("删除职工成功!\n");
break;
}
case 4: {
int ascending;
printf("请选择排序方式(1为升序,0为降序):");
scanf("%d", &ascending);
sortEmployee(&list, ascending);
printf("排序职工成功!\n");
break;
}
case 5: {
int id;
printf("请输入工号:");
scanf("%d", &id);
updateEmployee(&list, id);
printf("修改职工信息成功!\n");
break;
}
case 6: {
countEmployee(&list);
break;
}
case 7: {
printf("谢谢使用,再见!\n");
break;
}
default: {
printf("无效的操作,请重新输入!\n");
break;
}
}
} while (choice != 7);
//释放链表内存
Employee* current = list.head;
while (current != NULL) {
Employee* next = current->next;
free(current);
current = next;
}
return 0;
}
```
相关推荐
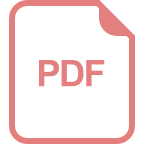
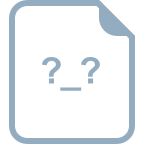
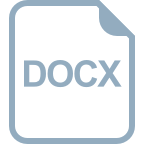
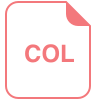
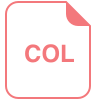
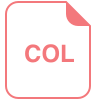
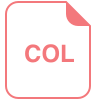
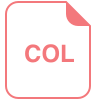










