请编写一个Java程序,要求输入一个整数n,然后输出n个随机字母,如果n不在1到10之间,则抛出一个自定义的异常InvalidInputException,并在主函数中进行捕获和处理。
时间: 2024-03-09 08:51:09 浏览: 26
好的,以下是Java程序的实现:
```java
import java.util.Random;
class InvalidInputException extends Exception {
public InvalidInputException(String message) {
super(message);
}
}
public class RandomLetters {
public static void main(String[] args) {
try {
int n = Integer.parseInt(args[0]);
if (n < 1 || n > 10) {
throw new InvalidInputException("Invalid input: n should be between 1 and 10.");
}
char[] letters = new char[n];
Random rand = new Random();
for (int i = 0; i < n; i++) {
letters[i] = (char) (rand.nextInt(26) + 'a');
}
System.out.println("Random letters: " + new String(letters));
} catch (NumberFormatException e) {
System.out.println("Invalid input: n should be an integer.");
} catch (InvalidInputException e) {
System.out.println(e.getMessage());
}
}
}
```
程序中使用了自定义的异常类InvalidInputException,当输入的n不在1到10之间时,会抛出该异常。主函数中通过try-catch语句捕获该异常,并输出错误信息。如果输入的n不是整数,则会抛出NumberFormatException异常。程序中使用Random类生成随机字母,并将它们存储在字符数组中,最后输出这些随机字母。
阅读全文
相关推荐
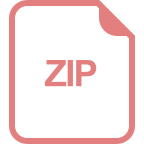
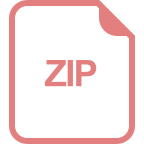
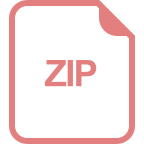




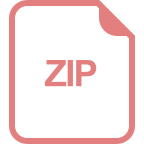
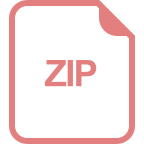
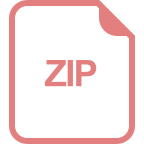
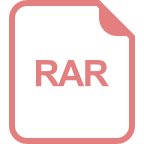
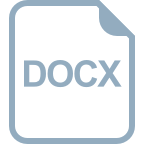
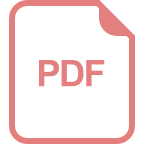
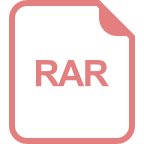