opencv怎么用填充轮廓实现热成像图片
时间: 2023-05-26 19:01:00 浏览: 262
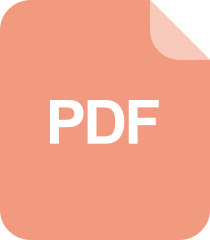
利用opencv进行图像处理
A:要实现热成像图片,需要使用OpenCV的填充函数对轮廓进行填充。以下是步骤:
1. 首先读取灰度图像并进行二值化处理。
``` python
import cv2
import numpy as np
img = cv2.imread('sample.png', 0)
ret, thresh = cv2.threshold(img, 100, 255, cv2.THRESH_BINARY)
```
2. 然后使用cv2.findContours()函数查找轮廓。
``` python
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
```
3. 然后使用cv2.drawContours()函数绘制轮廓。
``` python
img_contours = np.zeros(img.shape)
cv2.drawContours(img_contours, contours, -1, (255, 255, 255), 3)
```
4. 然后使用cv2.fillPoly()函数将轮廓填充。
``` python
cv2.fillPoly(img_contours, pts=[contours], color=(255, 255, 255))
```
5. 最后使用cv2.applyColorMap()函数将图像转换成热成像图。
``` python
heatmap = cv2.applyColorMap(img_contours, cv2.COLORMAP_HOT)
```
完整的代码如下:
``` python
import cv2
import numpy as np
img = cv2.imread('sample.png', 0)
ret, thresh = cv2.threshold(img, 100, 255, cv2.THRESH_BINARY)
contours, hierarchy = cv2.findContours(thresh, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
img_contours = np.zeros(img.shape)
cv2.drawContours(img_contours, contours, -1, (255, 255, 255), 3)
cv2.fillPoly(img_contours, pts=[contours], color=(255, 255, 255))
heatmap = cv2.applyColorMap(img_contours, cv2.COLORMAP_HOT)
cv2.imshow('Heatmap', heatmap)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
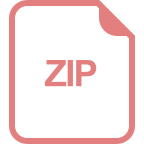
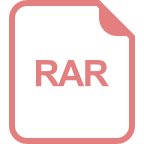

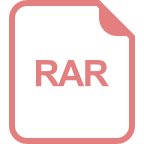
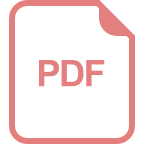
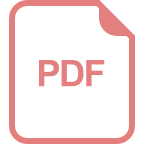
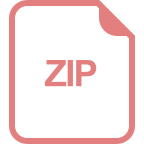
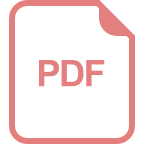
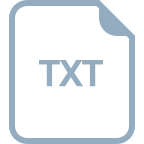
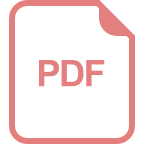
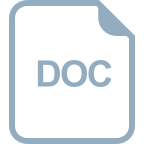
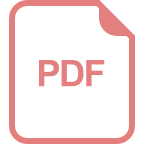