用C++设计一个数组类模板,该模板至少包含对数组元素的初始化、输出所有数组元素、输入所有数组元素、求某一个数组元素、修改某一个数组元素、求数组元素个数,对数组元素进行排序。设计一个自定义类并重载必要的输入输出运算符函数以实例化该模板测试其相关功能。
时间: 2024-01-13 19:05:47 浏览: 46
好的,我可以为你解答这个问题。
首先,我们可以定义一个数组类模板,包含以下成员函数:
```c++
template <typename T>
class Array {
private:
T* data; // 存储数组元素的指针
int size; // 数组大小
public:
// 构造函数
Array(int n = 0) {
size = n;
data = new T[size];
}
// 析构函数
~Array() {
delete[] data;
}
// 初始化数组元素
void init() {
for (int i = 0; i < size; i++) {
cout << "请输入第 " << i+1 << " 个元素:";
cin >> data[i];
}
}
// 输出所有数组元素
void output() {
for (int i = 0; i < size; i++) {
cout << data[i] << " ";
}
cout << endl;
}
// 输入所有数组元素
void input() {
for (int i = 0; i < size; i++) {
cout << "请输入第 " << i+1 << " 个元素:";
cin >> data[i];
}
}
// 求某一个数组元素
T get(int index) {
return data[index];
}
// 修改某一个数组元素
void set(int index, T value) {
data[index] = value;
}
// 求数组元素个数
int getSize() {
return size;
}
// 对数组元素进行排序
void sort() {
sort(data, data+size);
}
};
```
接着,我们可以定义一个自定义类 `Person`,重载必要的输入输出运算符函数,以实例化该模板测试其相关功能。
```c++
class Person {
private:
string name;
int age;
public:
// 构造函数
Person(string nm = "", int ag = 0) {
name = nm;
age = ag;
}
// 重载输入运算符
friend istream& operator>>(istream& is, Person& p) {
cout << "请输入姓名:";
is >> p.name;
cout << "请输入年龄:";
is >> p.age;
return is;
}
// 重载输出运算符
friend ostream& operator<<(ostream& os, const Person& p) {
os << "姓名:" << p.name << ",年龄:" << p.age << endl;
return os;
}
};
```
最后,我们可以在 `main` 函数中测试定义的数组类模板。
```c++
int main() {
// 测试数组类模板
Array<int> intArray(5);
intArray.init();
intArray.output();
cout << "第 3 个元素是:" << intArray.get(2) << endl;
intArray.set(2, 100);
intArray.output();
cout << "元素个数是:" << intArray.getSize() << endl;
intArray.sort();
intArray.output();
Array<double> doubleArray(3);
doubleArray.input();
doubleArray.output();
cout << "第 2 个元素是:" << doubleArray.get(1) << endl;
doubleArray.set(1, 3.14);
doubleArray.output();
cout << "元素个数是:" << doubleArray.getSize() << endl;
doubleArray.sort();
doubleArray.output();
Array<string> stringArray(4);
stringArray.init();
stringArray.output();
cout << "第 4 个元素是:" << stringArray.get(3) << endl;
stringArray.set(3, "Hello");
stringArray.output();
cout << "元素个数是:" << stringArray.getSize() << endl;
stringArray.sort();
stringArray.output();
Array<Person> personArray(2);
personArray.input();
personArray.output();
cout << "第 1 个元素是:" << personArray.get(0);
personArray.set(0, Person("Tom", 22));
personArray.output();
cout << "元素个数是:" << personArray.getSize() << endl;
personArray.sort();
personArray.output();
return 0;
}
```
这样,我们就实现了一个数组类模板,并用自定义类 `Person` 实例化了该模板进行测试。
相关推荐
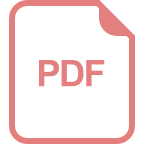













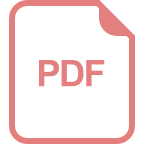