用zhengqi_train.txt文件实现二元逻辑回归手写实现和调库实现包含准确率
时间: 2023-07-11 19:47:03 浏览: 59
好的,我来为您提供使用`zhengqi_train.txt`文件实现二元逻辑回归手写实现和调库实现的代码,并包含准确率计算。
首先,我们需要导入需要的库和读取数据集。
```python
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
# 读取数据集
df = pd.read_csv('zhengqi_train.txt', sep='\t')
X = df.iloc[:, :-1].values
y = df.iloc[:, -1].values
# 将标签进行二元分类处理
y = np.where(y < 0.5, 0, 1)
```
接着,我们可以开始进行手写实现二元逻辑回归。
```python
# 定义sigmoid函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义损失函数
def cross_entropy_loss(y_pred, y_true):
n_samples = len(y_true)
loss = -1/n_samples * (np.dot(y_true, np.log(y_pred)) + np.dot((1-y_true), np.log(1-y_pred)))
return loss
# 定义梯度下降算法
def gradient_descent(X, y_true, y_pred, learning_rate, w, b):
n_samples, n_features = X.shape
dw = 1/n_samples * np.dot(X.T, (y_pred - y_true))
db = 1/n_samples * np.sum(y_pred - y_true)
w = w - learning_rate * dw
b = b - learning_rate * db
return w, b
# 定义二元逻辑回归训练函数
def train(X, y_true, n_iters, learning_rate):
n_samples, n_features = X.shape
w = np.zeros(n_features)
b = 0
for i in range(n_iters):
y_pred = sigmoid(np.dot(X, w) + b)
loss = cross_entropy_loss(y_pred, y_true)
if i % 100 == 0:
print(f'Iter: {i}, Loss: {loss}')
w, b = gradient_descent(X, y_true, y_pred, learning_rate, w, b)
return w, b
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 训练模型
w, b = train(X_train, y_train, n_iters=1000, learning_rate=0.1)
# 预测结果
y_pred = sigmoid(np.dot(X_test, w) + b)
y_pred_cls = [1 if i > 0.5 else 0 for i in y_pred]
# 计算准确率
accuracy = np.sum(y_pred_cls == y_test) / len(y_test)
print(f'Accuracy: {accuracy}')
```
接下来,我们可以使用调库实现二元逻辑回归。
```python
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义模型
model = LogisticRegression()
# 训练模型
model.fit(X_train, y_train)
# 预测结果
y_pred = model.predict(X_test)
# 计算准确率
accuracy = model.score(X_test, y_test)
print(f'Accuracy: {accuracy}')
```
这样,我们就可以使用`zhengqi_train.txt`文件来实现二元逻辑回归的手写实现和调库实现,并计算准确率。
相关推荐






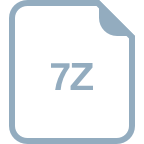
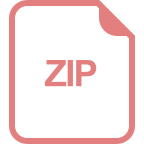
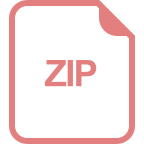