convert_from_bytes(f.read()),convert_from_bytes()函数的python代码是什么
时间: 2023-02-14 16:29:29 浏览: 140
`convert_from_bytes()`是一个自定义函数,具体实现取决于你的代码。
在给定的代码中,`f.read()`会读取文件`f`的内容,并将其转换为字节类型(byte type)。然后这个字节类型的值会作为参数传递给`convert_from_bytes()`函数,由此函数转换为另一种类型。
如果你想知道`convert_from_bytes()`函数的具体实现,需要查看该函数在代码中的定义。
相关问题
File "E:\code-study\coda\crossing\TEST.py", line 52, in <module> df["geometry"] = df["geometry"].apply(wkt.loads) File "C:\Users\GW00321286\.conda\envs\python_39\lib\site-packages\pandas\core\series.py", line 4771, in apply return SeriesApply(self, func, convert_dtype, args, kwargs).apply() File "C:\Users\GW00321286\.conda\envs\python_39\lib\site-packages\pandas\core\apply.py", line 1123, in apply return self.apply_standard() File "C:\Users\GW00321286\.conda\envs\python_39\lib\site-packages\pandas\core\apply.py", line 1174, in apply_standard mapped = lib.map_infer( File "pandas\_libs\lib.pyx", line 2924, in pandas._libs.lib.map_infer File "C:\Users\GW00321286\.conda\envs\python_39\lib\site-packages\shapely\wkt.py", line 22, in loads return shapely.from_wkt(data) File "C:\Users\GW00321286\.conda\envs\python_39\lib\site-packages\shapely\io.py", line 286, in from_wkt return lib.from_wkt(geometry, invalid_handler, **kwargs) TypeError: Expected bytes or string, got MultiLineString
根据错误消息,看起来是在使用 `wkt.loads` 函数时出现了错误。它期望的输入是字节或字符串,但实际上传入的是 MultiLineString 类型的几何对象。
要解决这个问题,您可以尝试使用 `wkb.loads` 函数,它接受二进制格式(WKB)的几何数据。可以通过将 `wkb.dumps` 函数应用于 MultiLineString 类型的几何对象来获取 WKB 格式的数据。以下是一种可能的解决方案:
```python
import geopandas as gpd
from shapely import wkb
# 读取Geopandas数据
gdf = gpd.read_file('your_data.geojson')
# 将MultiLineString几何对象转换为WKB格式
gdf['geometry'] = gdf['geometry'].apply(lambda x: wkb.dumps(x))
# 将WKB格式的几何数据转换回几何对象
gdf['geometry'] = gdf['geometry'].apply(lambda x: wkb.loads(x))
# 打印结果
print(gdf)
```
在这段代码中,我们首先将 MultiLineString 类型的几何对象转换为 WKB 格式,然后再将其转换回几何对象。请确保将 'your_data.geojson' 替换为您自己的 Geopandas 数据文件路径。
第33行报错:发生异常: error unpack requires a buffer of 12 bytes File "/Users/dingfengyan/工作/项目开发/哈理工/批量导出功能/binzcsv+feature.py", line 33, in convert_binary_to_csv float_data = struct.unpack("fff", binary_data) ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ File "/Users/dingfengyan/工作/项目开发/哈理工/批量导出功能/binzcsv+feature.py", line 20, in extract_zip_files convert_binary_to_csv(bin_data, output_file_path) File "/Users/dingfengyan/工作/项目开发/哈理工/批量导出功能/binzcsv+feature.py", line 11, in extract_and_convert_zip_files extract_zip_files(file_path, output_folder) File "/Users/dingfengyan/工作/项目开发/哈理工/批量导出功能/binzcsv+feature.py", line 68, in <module> extract_and_convert_zip_files(currentPath, currentPath) struct.error: unpack requires a buffer of 12 bytes
这个错误是由于在第33行的 `struct.unpack` 函数中,指定的格式字符串 `"fff"` 要求输入的 `binary_data` 长度必须为 12 字节,但实际上 `binary_data` 的长度可能小于 12 字节。这可能是由于您的 BIN 文件的格式不符合预期导致的。
为了解决这个问题,您可以在调用 `struct.unpack` 之前,添加一些检查来确保 `binary_data` 的长度足够。以下是修改后的代码示例:
```python
import os
import csv
import struct
import zipfile
def extract_and_convert_zip_files(folder_path, output_folder):
for root, dirs, files in os.walk(folder_path):
for file in files:
if file.endswith(".zip"):
file_path = os.path.join(root, file)
extract_zip_files(file_path, output_folder)
def extract_zip_files(file_path, output_folder):
with zipfile.ZipFile(file_path, "r") as zip_file:
for file_info in zip_file.infolist():
if file_info.filename.endswith(".bin"):
bin_data = zip_file.read(file_info.filename)
output_file_name = os.path.splitext(file_info.filename)[0] + "_original.csv"
output_file_path = os.path.join(output_folder, output_file_name)
convert_binary_to_csv(bin_data, output_file_path)
def convert_binary_to_csv(bin_data, output_file_path):
with open(output_file_path, "w", newline="") as csv_file:
csv_writer = csv.writer(csv_file)
csv_writer.writerow(["Column 1", "Column 2", "Column 3"])
pos = 0
while pos < len(bin_data):
if len(bin_data) - pos >= 12: # 检查剩余的数据是否足够
binary_data = bin_data[pos:pos+12] # 读取12字节数据
pos += 12
float_data = struct.unpack("fff", binary_data)
csv_writer.writerow(float_data)
else:
break # 如果剩余的数据不足12字节,跳出循环
# 指定文件夹路径和输出文件夹路径进行调用
extract_and_convert_zip_files("your_folder_path", "your_output_folder_path")
```
请将代码中的 "your_folder_path" 和 "your_output_folder_path" 替换为您实际的文件夹路径和输出文件夹路径。
在修改后的代码中,我们首先添加了一个检查,确保剩余的数据长度大于等于 12 字节才执行 `struct.unpack` 操作。如果剩余的数据长度不足 12 字节,就跳出循环,避免继续解析不完整的数据。
这样修改后,您应该能够处理 BIN 文件并将数据转换为 CSV 文件了。如果仍然遇到问题,请确保您的 BIN 文件的格式与代码中指定的结构一致,每个数据项都占用 4 字节。
阅读全文
相关推荐
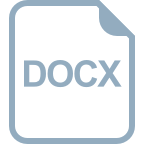
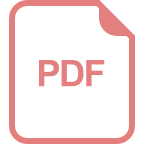
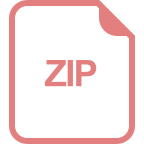
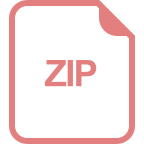
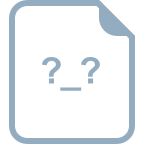
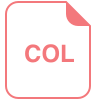
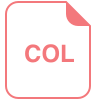
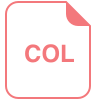
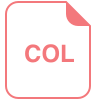
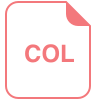
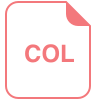
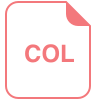
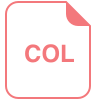
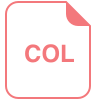

