java获取某天的结束时间 格式为yyyy-MM-dd HH:mm:ss
时间: 2023-08-31 17:39:01 浏览: 101
### 回答1:
可以通过以下代码获取某天的结束时间:
```java
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class Demo {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Calendar calendar = Calendar.getInstance();
calendar.setTime(new Date()); // 设置为当前时间
calendar.set(Calendar.HOUR_OF_DAY, 23); // 将小时数设置为23
calendar.set(Calendar.MINUTE, 59); // 将分钟数设置为59
calendar.set(Calendar.SECOND, 59); // 将秒数设置为59
String endTime = sdf.format(calendar.getTime()); // 格式化输出
System.out.println(endTime); // 输出结果
}
}
```
其中,使用 `Calendar` 类设置小时数、分钟数和秒数,最后使用 `SimpleDateFormat` 类格式化输出,得到结束时间的字符串表示。
### 回答2:
可以使用Java中的日期和时间类来获取某天的结束时间。具体步骤如下:
1. 首先,需要导入java.time包下的相关类,如下所示:
```
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
```
2. 然后,使用`LocalDate`类的`now()`方法获取当前日期,使用`LocalTime`类的`MAX`常量获取一天的最后时间。再将日期和时间合并为完整的日期时间对象:
```
LocalDate currentDate = LocalDate.now();
LocalDateTime endTime = LocalDateTime.of(currentDate, LocalTime.MAX);
```
3. 最后,使用`DateTimeFormatter`类来格式化日期时间对象为指定的格式:
```
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String endTimeString = endTime.format(formatter);
```
完整的代码如下:
```
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
LocalDate currentDate = LocalDate.now();
LocalDateTime endTime = LocalDateTime.of(currentDate, LocalTime.MAX);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String endTimeString = endTime.format(formatter);
System.out.println("结束时间:" + endTimeString);
}
}
```
以上代码将输出当前日期的结束时间,格式为"yyyy-MM-dd HH:mm:ss"。
### 回答3:
要获取某天的结束时间,可以使用Java中的`Calendar`和`SimpleDateFormat`类来实现。
首先,我们需要创建一个`Calendar`对象,并将日期设置为所需的某天。代码如下:
```java
Calendar calendar = Calendar.getInstance();
calendar.set(Calendar.YEAR, 年);
calendar.set(Calendar.MONTH, 月-1);
calendar.set(Calendar.DAY_OF_MONTH, 日);
```
以上的`年`、`月`和`日`分别代表所需日期的年份、月份和日期。需要注意的是,月份是从0开始计数的,所以如果要获取3月份的结束时间,需要将月份设置为2(即3-1)。
接下来,我们需要将时间设置为当天的最后一刻,即23:59:59。代码如下:
```java
calendar.set(Calendar.HOUR_OF_DAY, 23);
calendar.set(Calendar.MINUTE, 59);
calendar.set(Calendar.SECOND, 59);
```
然后,我们需要将时间格式化为指定的格式,即yyyy-MM-dd HH:mm:ss。代码如下:
```java
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String endTime = sdf.format(calendar.getTime());
```
最后,我们可以通过`endTime`变量获取到某天的结束时间,格式为yyyy-MM-dd HH:mm:ss。
完整的代码示例如下:
```java
import java.util.Calendar;
import java.text.SimpleDateFormat;
public class GetEndTime {
public static void main(String[] args) {
int year = 2022;
int month = 3;
int day = 15;
Calendar calendar = Calendar.getInstance();
calendar.set(Calendar.YEAR, year);
calendar.set(Calendar.MONTH, month-1);
calendar.set(Calendar.DAY_OF_MONTH, day);
calendar.set(Calendar.HOUR_OF_DAY, 23);
calendar.set(Calendar.MINUTE, 59);
calendar.set(Calendar.SECOND, 59);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String endTime = sdf.format(calendar.getTime());
System.out.println("某天的结束时间为:" + endTime);
}
}
```
以上代码可以得到某天的结束时间,输出为"某天的结束时间为:2022-03-15 23:59:59"。
阅读全文
相关推荐
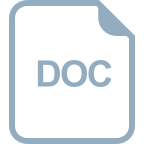
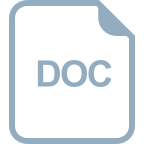
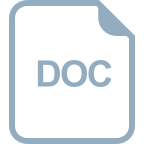


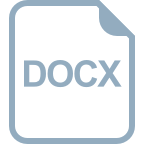
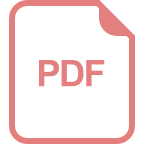










