c++ int to string
时间: 2023-12-07 16:35:06 浏览: 24
将 int 类型转换为 string 类型可以使用 C++ 标准库中的 to_string 函数。示例代码如下:
```c++
#include <iostream>
#include <string>
int main() {
int num = 123;
std::string str = std::to_string(num);
std::cout << str << std::endl;
return 0;
}
```
输出结果为:
```
123
```
相关问题
C++ int to string
To convert an integer to a string in C, you can use the sprintf() function. The sprintf() function formats and stores a series of characters and values in a string.
Here's an example:
```c
#include <stdio.h>
int main() {
int num = 123;
char str[20];
sprintf(str, "%d", num);
printf("The integer is: %d\n", num);
printf("The string is: %s\n", str);
return 0;
}
```
Output:
```
The integer is: 123
The string is: 123
```
In the above example, we declared an integer variable "num" and set it to 123. We also declared a character array "str" to store the string version of the integer.
We then used the sprintf() function to convert the integer to a string and store it in the "str" array. The first argument of sprintf() is the buffer to store the string, the second argument is the format string, and the third argument is the integer to be converted.
Finally, we printed both the integer and string versions of the variable to the console.
int to string c++
有几种方法可以将int类型转换为string类型。其中一种方法是使用to_string函数。这个函数可以将一个整数转换为对应的字符串。例如,如果你有一个整数变量i,你可以使用to_string(i)来将其转换为字符串。另外一种方法是使用stringstream。你可以创建一个stringstream对象,然后使用<<操作符将整数插入到stringstream中,最后使用.str()函数获取转换后的字符串。例如,如果你有一个整数变量i,你可以使用stringstream ss; ss << i; string s = ss.str(); 将其转换为字符串。还有一种方法是使用sprintf函数。这个函数可以将一个格式化的字符串写入到一个字符数组中。你可以创建一个足够大的字符数组,然后使用sprintf函数将整数格式化成字符串并存储在数组中。例如,如果你有一个整数变量i和一个字符数组buffer,你可以使用sprintf(buffer, "%d", i); 将其转换为字符串。请注意,这里的%d是用来指定整数的格式化字符串。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [C++ int与string的转化](https://blog.csdn.net/weixin_34378767/article/details/85658812)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
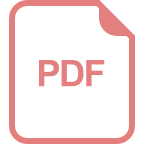












